写一个python代码,实现求函数f(x)=x2+5的最大值,变量x取0到31之间的整数值。通过遗传算法确定函数最大值
时间: 2024-05-06 20:20:21 浏览: 144
这里是一个可能的实现,使用Python的遗传算法库DEAP:
```python
import random
from deap import algorithms, base, creator, tools
# 定义适应度函数
def evaluate(individual):
x = individual[0]
return x ** 5,
# 定义个体和种群
creator.create("FitnessMax", base.Fitness, weights=(1.0,))
creator.create("Individual", list, fitness=creator.FitnessMax)
toolbox = base.Toolbox()
toolbox.register("attr_x", random.randint, 0, 31)
toolbox.register("individual", tools.initRepeat, creator.Individual, toolbox.attr_x, n=1)
toolbox.register("population", tools.initRepeat, list, toolbox.individual)
# 定义遗传算法的参数
toolbox.register("evaluate", evaluate)
toolbox.register("mate", tools.cxTwoPoint)
toolbox.register("mutate", tools.mutUniformInt, low=0, up=31, indpb=0.1)
toolbox.register("select", tools.selTournament, tournsize=3)
population = toolbox.population(n=100)
NGEN = 50
CXPB = 0.5
MUTPB = 0.2
# 运行遗传算法
for gen in range(NGEN):
offspring = algorithms.varAnd(population, toolbox, cxpb=CXPB, mutpb=MUTPB)
fits = toolbox.map(toolbox.evaluate, offspring)
for fit, ind in zip(fits, offspring):
ind.fitness.values = fit
population = toolbox.select(offspring, k=len(population))
best_ind = tools.selBest(population, k=1)[0]
print("最大值: %s" % (best_ind[0] ** 5,))
```
这个代码使用了基本的遗传算法框架,其中定义了适应度函数、个体和种群的属性,以及遗传算法的参数和操作。在主循环中,使用DEAP的`algorithms.varAnd`函数进行种群的进化,同时计算每个个体的适应度,并使用DEAP的`tools.selBest`函数选择最佳个体。最终输出最大值。
阅读全文
相关推荐
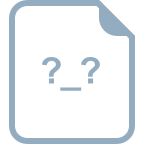
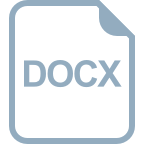
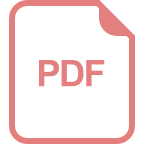
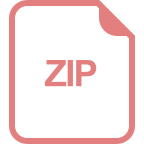












