编写程序实现如下功能从argv[1]所指定的文件中读取内容,依次写到管道/home/linux/myfifo中。
时间: 2024-01-12 10:05:08 浏览: 102
好的,以下是一个简单的C语言程序,可以实现你所需的功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
int main(int argc, char *argv[]) {
if (argc != 2) {
printf("Usage: %s filename\n", argv[0]);
exit(1);
}
// 打开文件
int fd = open(argv[1], O_RDONLY);
if (fd < 0) {
perror("open file error");
exit(1);
}
// 打开管道
int fifo_fd = open("/home/linux/myfifo", O_WRONLY);
if (fifo_fd < 0) {
perror("open fifo error");
exit(1);
}
// 读取文件内容并写入管道
char buf[1024];
int n;
while ((n = read(fd, buf, sizeof(buf))) > 0) {
write(fifo_fd, buf, n);
}
// 关闭文件和管道
close(fd);
close(fifo_fd);
return 0;
}
```
你可以将上述代码保存为一个名为 `copy_to_fifo.c` 的文件,然后使用以下命令编译并执行程序:
```
gcc copy_to_fifo.c -o copy_to_fifo
./copy_to_fifo filename
```
其中,`filename` 为你需要读取的文件名。执行程序后,该程序会将文件内容依次写入管道 `/home/linux/myfifo` 中。
相关推荐
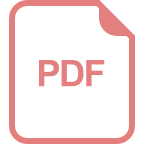
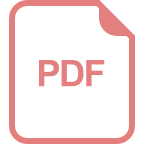
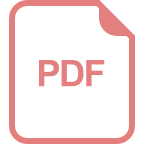














