怎么从文件中读取内容到数据库中用C写
时间: 2024-05-11 17:15:52 浏览: 10
您可以使用标准C库中的文件操作函数来读取文件内容,并使用数据库API将读取的内容写入数据库中。具体实现取决于您使用的数据库和文件格式。以下是一个基本的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sqlite3.h> // SQLite3 数据库API
#define MAX_LINE_LENGTH 256 // 文件每行最大长度
int main(int argc, char* argv[]) {
// 打开文件
FILE* fp = fopen("data.txt", "r");
if (!fp) {
printf("Failed to open file!\n");
exit(1);
}
// 打开数据库(使用 SQLite3)
sqlite3* db;
int rc = sqlite3_open("database.db", &db);
if (rc != SQLITE_OK) {
printf("Failed to open database: %s\n", sqlite3_errmsg(db));
exit(1);
}
// 创建数据表
char* sql = "CREATE TABLE IF NOT EXISTS data (id INTEGER PRIMARY KEY, content TEXT);";
rc = sqlite3_exec(db, sql, NULL, NULL, NULL);
if (rc != SQLITE_OK) {
printf("Failed to create table: %s\n", sqlite3_errmsg(db));
exit(1);
}
// 逐行读取文件并插入数据库
char line[MAX_LINE_LENGTH];
int id = 0;
while (fgets(line, MAX_LINE_LENGTH, fp)) {
// 去掉行末的换行符
int len = strlen(line);
if (line[len - 1] == '\n') {
line[len - 1] = '\0';
}
// 构造插入语句并执行
char* stmt = sqlite3_mprintf("INSERT INTO data (id, content) VALUES (%d, '%q')", id++, line);
rc = sqlite3_exec(db, stmt, NULL, NULL, NULL);
if (rc != SQLITE_OK) {
printf("Failed to insert data: %s\n", sqlite3_errmsg(db));
exit(1);
}
sqlite3_free(stmt);
}
// 关闭文件和数据库
fclose(fp);
sqlite3_close(db);
return 0;
}
```
上述代码使用标准C库中的 `fopen` 函数打开文件,然后逐行读取文件内容,使用 SQLite3 数据库API将每行内容插入到名为 `data` 的数据表中。您可以根据需要修改表名和列名等参数。
相关推荐
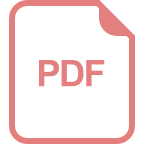
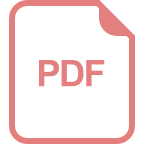
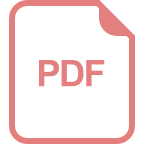
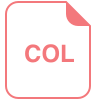













