如何从界面读取图片到数据库中
时间: 2024-01-24 11:17:16 浏览: 110
要从界面读取图片并将其保存到数据库中,您需要执行以下步骤:
1. 在您的界面上添加一个“选择文件”按钮,当用户单击该按钮时,它将打开文件选取器对话框。
2. 在对话框中选择要保存到数据库中的图像,并使用文件选取器返回文件的路径。
3. 使用选定的文件路径从磁盘读取图像数据。
4. 将图像数据转换为二进制格式。
5. 将二进制数据插入到数据库中的图像字段中。
以下是一个示例代码片段,演示如何实现此过程:
```python
import tkinter as tk
from tkinter import filedialog
import mysql.connector
from mysql.connector import Error
from PIL import Image
# 创建一个 Tkinter 窗口
window = tk.Tk()
# 创建一个函数来打开文件选择器对话框并返回所选文件的路径
def select_file():
file_path = filedialog.askopenfilename()
return file_path
# 创建一个函数来将文件读取为二进制格式并将其保存到数据库中
def save_image_to_database():
try:
# 连接到 MySQL 数据库
connection = mysql.connector.connect(
host="localhost",
user="root",
password="password",
database="database_name"
)
# 获取文件路径
file_path = select_file()
# 打开图像文件并将其转换为二进制格式
with open(file_path, "rb") as f:
binary_data = f.read()
# 将二进制数据插入到数据库中
cursor = connection.cursor()
insert_query = "INSERT INTO images (image_data) VALUES (%s)"
cursor.execute(insert_query, (binary_data,))
connection.commit()
cursor.close()
except Error as e:
print("Error while connecting to MySQL", e)
finally:
# 关闭数据库连接
if (connection.is_connected()):
connection.close()
# 创建一个“选择文件”按钮,并将其绑定到 select_file 函数上
select_button = tk.Button(window, text="选择文件", command=select_file)
select_button.pack()
# 创建一个“保存到数据库”按钮,并将其绑定到 save_image_to_database 函数上
save_button = tk.Button(window, text="保存到数据库", command=save_image_to_database)
save_button.pack()
# 运行 Tkinter 窗口
window.mainloop()
```
请注意,此示例仅是一个基本的框架,您可能需要根据您的具体情况进行修改和调整。
阅读全文
相关推荐
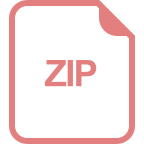
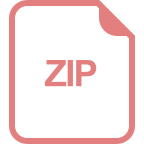
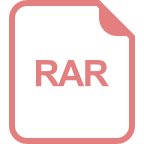
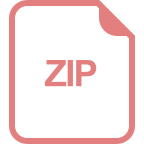
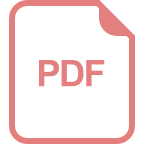
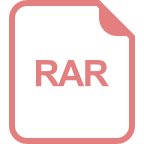
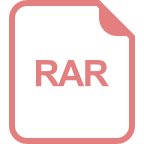
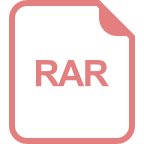
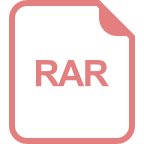
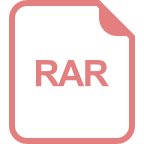
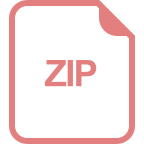
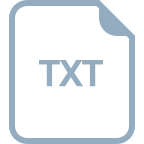
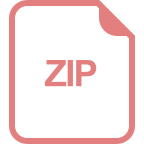
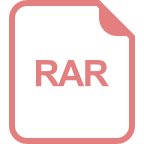
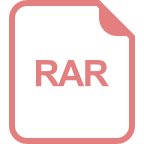
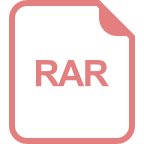