#include <iostream> using namespace std; #include<string> #include"math.h" template<class T> class vlist//线性表抽象类 { public: virtual void in(T &e)=0; virtual T out()=0; }; template<class T> class Element//线性表存储元素 { public: T data; Element<T> *next; Element(){} }; //在beign和end之间完成SStack和Quene类的定义及实现,其继承vlist /***************begin****************/ /***************end******************/ int main() { vlist<int> *intp; vlist<string> *strp; SStack<int> ints; Quene<int> intq; SStack<string> strs; Quene<string> strq; intp=&ints; int t=2; intp->in(t); t=3; intp->in(t); t=4; intp->in(t); for(int i=0;i<3;i++) cout<<intp->out(); intp=&intq; t=2; intp->in(t); t=3; intp->in(t); t=4; intp->in(t); for(int i=0;i<3;i++) cout<<intp->out(); strp=&strs; string str; cout<<endl; for(int i=0;i<3;i++) { cin>>str; strp->in(str); } for(int i=0;i<3;i++) cout<<strp->out()<<" "; cout<<endl; strp=&strq; for(int i=0;i<3;i++) { cin>>str; strp->in(str); } for(int i=0;i<3;i++) cout<<strp->out()<<" "; }
时间: 2023-08-06 21:06:51 浏览: 103
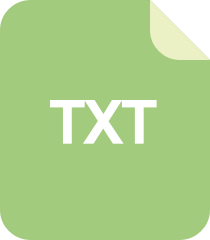
#include_iostream.h_

以下是完整的代码,其中包括了SStack和Quene类的定义及实现:
```c++
#include <iostream>
#include <string>
#include <cmath>
using namespace std;
template<class T>
class vlist // 线性表抽象类
{
public:
virtual void in(T &e) = 0;
virtual T out() = 0;
};
template<class T>
class Element // 线性表存储元素
{
public:
T data;
Element<T> *next;
Element() {}
};
template<class T>
class SStack : public vlist<T> // 栈类
{
private:
Element<T> *top;
public:
SStack() { top = NULL; }
void in(T &e) {
Element<T> *p = new Element<T>;
p->data = e;
p->next = top;
top = p;
}
T out() {
if (top == NULL) {
cerr << "Stack is empty!" << endl;
exit(1);
}
T e = top->data;
Element<T> *p = top;
top = top->next;
delete p;
return e;
}
};
template<class T>
class Quene : public vlist<T> // 队列类
{
private:
Element<T> *front, *rear;
public:
Quene() { front = rear = NULL; }
void in(T &e) {
Element<T> *p = new Element<T>;
p->data = e;
p->next = NULL;
if (rear == NULL) {
front = rear = p;
} else {
rear->next = p;
rear = p;
}
}
T out() {
if (front == NULL) {
cerr << "Quene is empty!" << endl;
exit(1);
}
T e = front->data;
Element<T> *p = front;
front = front->next;
if (front == NULL) {
rear = NULL;
}
delete p;
return e;
}
};
int main() {
vlist<int> *intp;
vlist<string> *strp;
SStack<int> ints;
Quene<int> intq;
SStack<string> strs;
Quene<string> strq;
intp = &ints;
int t = 2;
intp->in(t);
t = 3;
intp->in(t);
t = 4;
intp->in(t);
for (int i = 0; i < 3; i++) {
cout << intp->out();
}
intp = &intq;
t = 2;
intp->in(t);
t = 3;
intp->in(t);
t = 4;
intp->in(t);
for (int i = 0; i < 3; i++) {
cout << intp->out();
}
strp = &strs;
string str;
cout << endl;
for (int i = 0; i < 3; i++) {
cin >> str;
strp->in(str);
}
for (int i = 0; i < 3; i++) {
cout << strp->out() << " ";
}
cout << endl;
strp = &strq;
for (int i = 0; i < 3; i++) {
cin >> str;
strp->in(str);
}
for (int i = 0; i < 3; i++) {
cout << strp->out() << " ";
}
return 0;
}
```
在该代码中,我们先定义了vlist类作为线性表的抽象类,其中包括了in()方法和out()方法,用于元素的插入和删除。然后分别定义了SStack类和Quene类,它们分别继承自vlist类,实现了栈和队列的功能。其中,SStack类使用了链表来实现,top指向栈顶元素;Quene类也使用了链表来实现,front指向队首元素,rear指向队尾元素。
在主函数中,我们定义了四个线性表对象:ints、intq、strs、strq,分别为int类型的栈、int类型的队列、string类型的栈、string类型的队列。然后通过向它们添加元素和删除元素,测试它们的功能是否正确。
注意:在定义类模板时,需要在类名后面加上<class T>或者<typename T>,来定义模板参数类型。在定义成员函数时也需要加上<class T>或者<typename T>,来指明这是一个类模板的成员函数。同时,由于vlist类是一个抽象类,因此在定义成员函数时需要加上=0,来表示这些成员函数都是纯虚函数,需要在子类中实现。
阅读全文
相关推荐
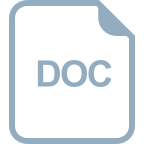
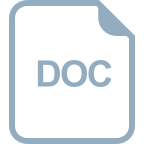
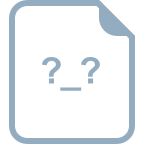
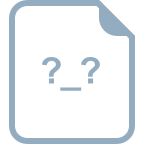
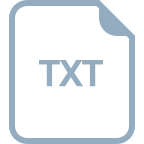
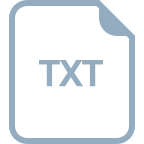
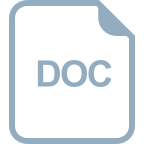
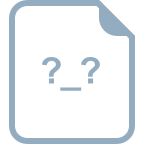
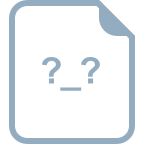
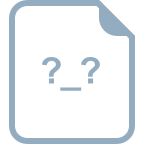
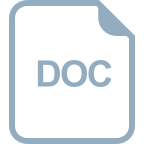
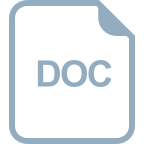
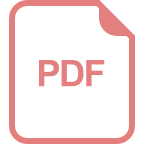
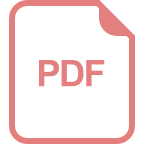
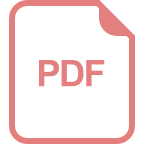
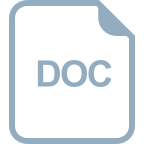