请用pytorch设计一个输入维度为2d的自注意力算子
时间: 2024-01-24 10:17:44 浏览: 21
好的,以下是一个使用PyTorch实现的2D自注意力算子:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class SelfAttention2d(nn.Module):
def __init__(self, in_channels):
super(SelfAttention2d, self).__init__()
self.in_channels = in_channels
self.theta = nn.Conv2d(in_channels, in_channels // 8, kernel_size=1, stride=1, padding=0, bias=False)
self.phi = nn.Conv2d(in_channels, in_channels // 8, kernel_size=1, stride=1, padding=0, bias=False)
self.g = nn.Conv2d(in_channels, in_channels // 2, kernel_size=1, stride=1, padding=0, bias=False)
self.out = nn.Conv2d(in_channels // 2, in_channels, kernel_size=1, stride=1, padding=0, bias=False)
self.gamma = nn.Parameter(torch.zeros(1))
def forward(self, x):
batch_size, channels, height, width = x.size()
theta = self.theta(x).view(batch_size, -1, height * width).permute(0, 2, 1)
phi = self.phi(x).view(batch_size, -1, height * width)
g = self.g(x).view(batch_size, -1, height * width).permute(0, 2, 1)
energy = torch.bmm(theta, phi)
attention = F.softmax(energy, dim=-1)
out = torch.bmm(g, attention.permute(0, 2, 1))
out = out.permute(0, 2, 1).contiguous().view(batch_size, -1, height, width)
out = self.gamma * self.out(out) + x
return out
```
这个自注意力算子的输入维度为2D,即 `(batch_size, channels, height, width)`。它将输入张量 `x` 分别传递到三个卷积层中,得到三个特征图 `theta`, `phi` 和 `g`。然后,通过矩阵乘法计算注意力矩阵,使用softmax函数进行归一化,并将其应用于 `g`。最后,我们将输出特征图 `out` 与输入张量 `x` 相加,并使用可学习的参数 `gamma` 进行缩放。
可以将这个自注意力算子与其他模块结合使用,例如在卷积神经网络中嵌入自注意力机制,以提高模型的性能。
相关推荐
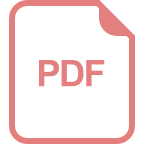














