用c++编写生命游戏,可以定义类Automaton对仿真建模, 也可以暂停和恢复仿真。
时间: 2024-03-18 21:38:38 浏览: 23
好的,我可以为您介绍一下用 C++ 编写生命游戏的实现方法。
首先,可以定义一个类 `Automaton` 来对生命游戏进行建模。在这个类中,需要定义一个二维数组来表示细胞的状态,1 表示细胞存活,0 表示细胞死亡。同时,可以定义一个 `display()` 函数来展示当前的细胞状态。
然后,可以实现生命游戏的规则。在每一轮仿真中,需要对每个细胞的状态进行判断,并更新它的状态。这个判断规则可以根据生命游戏的规则来实现。
最后,可以实现暂停和恢复仿真的功能。可以定义一个 `pause()` 函数来暂停仿真,同时定义一个 `resume()` 函数来恢复仿真。
下面是一个简单的生命游戏的代码示例:
```c++
#include <iostream>
#include <chrono>
#include <thread>
class Automaton {
public:
Automaton(int size) {
// 初始化细胞状态
for(int i=0; i<size; i++) {
for(int j=0; j<size; j++) {
cells[i][j] = rand() % 2;
}
}
}
void display() {
// 展示当前细胞状态
for(int i=0; i<size; i++) {
for(int j=0; j<size; j++) {
std::cout << cells[i][j] << " ";
}
std::cout << std::endl;
}
}
void simulate() {
while(true) {
// 判断每个细胞的状态
for(int i=0; i<size; i++) {
for(int j=0; j<size; j++) {
int neighbors = countNeighbors(i, j);
if(cells[i][j] == 1) {
if(neighbors < 2 || neighbors > 3) {
cells[i][j] = 0;
}
} else {
if(neighbors == 3) {
cells[i][j] = 1;
}
}
}
}
// 展示当前细胞状态
display();
// 暂停仿真
std::this_thread::sleep_for(std::chrono::milliseconds(500));
}
}
void pause() {
// 暂停仿真
paused = true;
}
void resume() {
// 恢复仿真
paused = false;
}
private:
int size = 10;
int cells[10][10];
bool paused = false;
int countNeighbors(int i, int j) {
// 计算一个细胞周围的存活细胞数量
int count = 0;
for(int x=i-1; x<=i+1; x++) {
for(int y=j-1; y<=j+1; y++) {
if(x >= 0 && x < size && y >= 0 && y < size && !(x == i && y == j)) {
count += cells[x][y];
}
}
}
return count;
}
};
int main() {
Automaton a(10);
a.simulate();
return 0;
}
```
这个示例代码中, `Automaton` 类实现了生命游戏的建模。在 `simulate()` 函数中,每次循环都会判断每个细胞的状态,并更新它的状态。同时,使用 `std::this_thread::sleep_for(std::chrono::milliseconds(500));` 函数来暂停仿真。在 `pause()` 和 `resume()` 函数中,可以实现暂停和恢复仿真的功能。
相关推荐
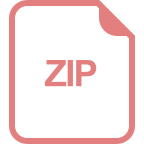
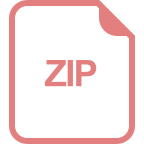
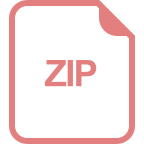














