def main(): pygame.init() screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT)) pygame.display.set_caption('贪吃蛇') font1 = pygame.font.SysFont('SimHei', 24) font2 = pygame.font.Font(None, 72) fwidth, fheight = font2.size('GAME OVER') b = True snake = init_snake() food = create_food(snake) food_style = get_food_style() pos = (1, 0) game_over = True start = False score = 0 orispeed = 0.5 speed = orispeed last_move_time = None pause = False
时间: 2024-04-27 15:24:15 浏览: 12
这段代码定义了一个名为`main`的函数,它没有参数。函数的作用是启动贪吃蛇游戏的主程序,包括初始化游戏界面、生成贪吃蛇和食物、控制游戏运行等。
具体实现过程如下:
1. 初始化Pygame模块。
2. 创建一个`SCREEN_WIDTH`宽、`SCREEN_HEIGHT`高的游戏界面,并设置标题为“贪吃蛇”。
3. 创建两个字体对象`font1`和`font2`,分别用于在游戏界面上显示得分和游戏结束文字。
4. 获取游戏结束文字的宽度和高度。
5. 定义一些游戏变量,包括贪吃蛇、食物、食物样式、移动方向、游戏是否结束、游戏是否开始、得分、初始速度、当前速度、上一次移动时间、是否暂停等。
6. 进入游戏循环,不断更新游戏状态并更新游戏界面,直到游戏结束。
7. 在游戏循环中,处理Pygame的事件队列,包括键盘按键和关闭窗口事件。
8. 如果游戏已经开始,根据当前时间和上一次移动时间的差值,更新贪吃蛇的位置和得分。
9. 如果贪吃蛇吃到了食物,更新得分和贪吃蛇身体,并生成新的食物。
10. 如果贪吃蛇碰到了边界或者自身身体,游戏结束,并显示游戏结束文字和得分。
11. 在游戏界面上显示贪吃蛇、食物、得分和暂停状态等。
12. 控制游戏运行速度和暂停状态。
相关问题
import pygame # 初始化Pygame pygame.init() # 设置窗口大小和标题 screen_width, screen_height = 600, 800 screen = pygame.display.set_mode((screen_width, screen_height)) pygame.display.set_caption("Tetris Game") class Block: def __init__(self, x, y, color): self.x = x self.y = y self.color = color self.shape = random.choice(shapes) self.rotation = 0 def move_down(self): self.y += 1 def move_left(self): self.x -= 1 def move_right(self): self.x += 1 def rotate(self): self.rotation = (self.rotation + 1) % len(self.shape) def main(): # 创建方块 block = Block(5, 0, random.choice(colors)) # 循环标志位 running = True # 游戏主循环 while running: # 事件处理 for event in pygame.event.get(): if event.type == pygame.QUIT: running = False # 绘制背景 screen.fill((255, 255, 255)) # 绘制方块 draw_block(block) # 更新屏幕 pygame.display.update() # 方块下落 block.move_down() # 检查方块是否到达底部 if block.y >= screen_height / block_size or check_collision(block): # 方块到达底部,创建新的方块 block = Block(5, 0, random.choice(colors)) # 检查是否有一行或多行方块被消除 remove_lines() # 延时 pygame.time.delay(100) def remove_lines(): global score lines = 0 for y in range(screen_height // block_size): if check_line(y): lines += 1 for x in range(screen_width // block_size): for i in range(len(blocks)): if blocks[i].x == x and blocks[i].y == y: del blocks[i] break if lines > 0: score += lines * 10 def draw_score(): font = pygame.font.Font(None, 36) score_text = font.render("Score: " + str(score), True, (0, 0, 0)) screen.blit(score_text, (10, 10))的系统概述
这段代码是一个使用Pygame库编写的俄罗斯方块游戏的主要部分,包括方块类的定义和移动方法,主循环的实现,方块下落和消除行的检查,以及得分的计算和显示。在主循环中,屏幕被填充为白色,方块被绘制在屏幕上,然后屏幕被更新,方块下落并检查是否到达底部或与其他方块碰撞,如果到达底部则创建新的方块。如果有一行或多行方块被消除,则得分增加。最后,得分被绘制在屏幕上。
我的程序是这样import sys import pygame class BlueSky: '''a blue sky''' def __init__(self) -> None: '''init''' pygame.init() self.screen = pygame.display.set_mode((1200, 800)) pygame.display.set_caption('test - blue sky') self.screen_rect = self.screen.get_rect() self.bg_color = (0, 20, 255) def run_game(self): '''start the game''' while True: for event in pygame.event.get(): if event.type == pygame.QUIT: sys.exit() pygame.display.flip() if __name__ == '__main__': ai = BlueSky() ai.run_game(),这里pygame.display设置为1200X800,用了pyautogui后,屏幕会自动缩小。这是我不希望的。
如果你不想让屏幕缩小,你可以在程序中禁用pyautogui的自动缩放功能。可以通过以下代码实现:
```
import pyautogui
pyautogui.PAUSE = 0.5 # 设置延迟时间为0.5秒
pyautogui.FAILSAFE = True # 打开pyautogui的安全防护措施
# 禁用自动缩放功能
pyautogui.displaySize = (pyautogui.size().width, pyautogui.size().height)
pyautogui.PAUSE = 0.5
# 在此之后的pyautogui操作将不会缩放屏幕
```
这样,程序中使用pyautogui的操作不会再缩放屏幕。
相关推荐
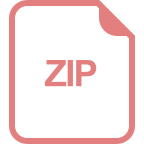
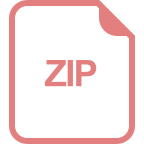












