import pygame import sys from pygame.locals import * from robomaster import * import cv2 if __name__ == '__main__': # 如果本地IP 自动获取不正确,手动指定本地IP地址 # robomaster.config.LOCAL_IP_STR = "192.168.2.20" ep_robot = robot.Robot() # 指定连接方式为AP 直连模式 ep_robot.initialize(conn_type='ap') version = ep_robot.get_version() print("Robot version: {0}".format(version)) def show_video(): # 获取机器人第一视角图像帧 img = ep_robot.camera.read_cv2_image(strategy="newest") # 转换图像格式,转换为pygame的surface对象 # if img.any(): img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) img = cv2.transpose(img) # 行列互换 img = pygame.surfarray.make_surface(img) screen.blit(img, (0, 0)) # 绘制图象 pygame.init() screen_size = width, height = 1280, 720 screen = pygame.display.set_mode(screen_size) ep_robot.camera.start_video_stream(display=False) pygame.time.wait(100) ep_robot.led.set_led(comp="all", r=255, g=255, b=255) # 亮绿灯 clock = pygame.time.Clock() while True: clock.tick(25) # 将帧数设置为25帧 for event in pygame.event.get(): if event.type == QUIT: ep_robot.close() pygame.quit() sys.exit() show_video() pygame.display.update()
时间: 2024-04-09 12:30:41 浏览: 13
这段代码是一个使用pygame和robomaster库进行机器人控制显示视频的示例代码。代码中首先导入了所需的库,然后创建了一个RobMaster机器人实例初始化连接方式为AP连模式。接下来获取机器人的版本信息并打印出来。
然后定义了一个名为show_video的函数,用于显示机器人的第一视角图像。在函数中,首先从机器人摄像头读取最新的图像帧,并转换为pygame的surface对象。然后将图像绘制到屏幕上。
着使用pygame进行初始化,并设置屏幕大小为1280x720。然后启动机器人的视频流,并等待100毫秒。同时,设置机器人的LED灯为全亮白色。
接下来进入主循环,其中使用clock对象设置帧率为25帧。在循环中,处理pygame的事件,当检测到QUIT事件时,关闭机器人连接、退出pygame并终止程序。然后调用show_video函数显示机器人视频,并更新屏幕显示。循环会不断重复执行,直到程序被终止。
请注意,这只是代码的一部分,可能还有其他部分未包含在内。如果你有关于这段代码的任何问题,请随时提问。
相关问题
import pygame import time import sys from pygame.locals import *
您好!这段代码是导入了Pygame游戏库,以及一些常用的模块和变量,其中包括:
- pygame:这是Pygame库的主模块,我们需要使用其中的函数和类来创建游戏。
- time:这个模块提供了一个接口来处理时间,可以用来控制游戏的速度、延迟等。
- sys:这个模块提供了一些函数和变量,可以用来控制Python解释器本身的一些属性和行为。
- pygame.locals:这个模块包含了Pygame库中常用的一些常量和枚举类型,可以用来表示键盘、鼠标、窗口等各种事件。
需要注意的是,这段代码只是导入了Pygame库,并没有实际创建游戏或者进行任何操作。如果您想要了解如何使用Pygame库来创建游戏,请参考相关的教程或者文档。
import random import sys import time import pygame from pygame.locals import * from collections import deque
您的代码有语法错误,应该将多个`import`语句分开写,每个`import`语句后面只能跟一个模块名或变量名。可以将代码修改为以下形式:
```python
import random
import sys
import time
import pygame
from pygame.locals import *
from collections import deque
```
这样就可以分别导入`random`、`sys`、`time`、`pygame`、`pygame.locals`和`collections`模块中的内容,方便我们在代码中使用它们。其中`from pygame.locals import *`表示从`pygame`模块中导入所有常量和函数,可以直接使用,而不需要加上模块名。`from collections import deque`表示从`collections`模块中只导入`deque`类。
相关推荐
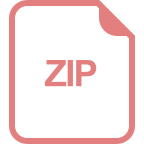













