PYTHON ANN for regression code
时间: 2024-05-12 22:20:32 浏览: 16
Here is an example code for building an Artificial Neural Network (ANN) model using Python for regression analysis:
```
# import required libraries
import numpy as np
import pandas as pd
import tensorflow as tf
from tensorflow import keras
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.metrics import r2_score, mean_squared_error
# load dataset
dataset = pd.read_csv('dataset.csv')
# split dataset into features and target variable
X = dataset.iloc[:, :-1].values
y = dataset.iloc[:, -1].values
# split dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
# scale features using StandardScaler
sc = StandardScaler()
X_train = sc.fit_transform(X_train)
X_test = sc.transform(X_test)
# build ANN model
model = keras.Sequential([
keras.layers.Dense(64, activation='relu', input_shape=(X_train.shape[1],)),
keras.layers.Dense(32, activation='relu'),
keras.layers.Dense(1)
])
# compile model
model.compile(optimizer='adam', loss='mean_squared_error')
# train model
model.fit(X_train, y_train, epochs=100, batch_size=32, verbose=0)
# evaluate model
y_pred = model.predict(X_test)
r2 = r2_score(y_test, y_pred)
mse = mean_squared_error(y_test, y_pred)
print('R2 score:', r2)
print('Mean squared error:', mse)
```
In this code, we first import necessary libraries such as numpy, pandas, and tensorflow. We then load the dataset and split it into features (X) and target variable (y) using pandas. We further split the dataset into training and testing sets using sklearn's train_test_split function. We also standardize the features using sklearn's StandardScaler.
Next, we build an ANN model using keras.Sequential and add three layers: two hidden layers with 64 and 32 neurons and the ReLU activation function, and an output layer with one neuron. We compile the model using the Adam optimizer and the mean squared error loss function, and train it for 100 epochs with a batch size of 32.
Finally, we evaluate the model by predicting the target variable for the testing set and calculating the R2 score and mean squared error using sklearn's r2_score and mean_squared_error functions, respectively.
相关推荐
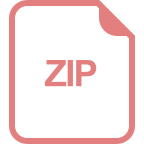
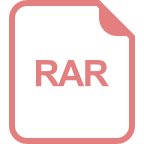
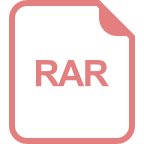














