可以帮我写一个根据mysql与Java的仓库管理系统吗
时间: 2024-01-22 09:02:24 浏览: 140
好的,我可以帮您提供一个简单的Java仓库管理系统的代码框架,使用MySQL数据库进行数据存储和管理。请注意,这只是一个基本的框架,您可能需要根据您的具体需求进行一些修改和扩展。
首先,您需要在MySQL数据库中创建一个名为“inventory”的数据库,并创建一个名为“products”的表,该表包含以下字段:id (int, 自增长), name (varchar), description (varchar), quantity (int), price (float)。
接下来,您需要在Java中创建一个名为“Product”的类,表示存储在仓库中的产品。示例代码如下:
```
public class Product {
private int id;
private String name;
private String description;
private int quantity;
private float price;
public Product(int id, String name, String description, int quantity, float price) {
this.id = id;
this.name = name;
this.description = description;
this.quantity = quantity;
this.price = price;
}
// Getters and setters
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public float getPrice() {
return price;
}
public void setPrice(float price) {
this.price = price;
}
}
```
然后,您需要创建一个名为“Inventory”的类,该类将执行与MySQL数据库的交互和数据管理。示例代码如下:
```
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class Inventory {
private Connection connection;
public Inventory() {
try {
Class.forName("com.mysql.cj.jdbc.Driver");
connection = DriverManager.getConnection("jdbc:mysql://localhost/inventory", "username", "password");
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
}
}
public void addProduct(Product product) {
try {
PreparedStatement statement = connection.prepareStatement("INSERT INTO products (name, description, quantity, price) VALUES (?, ?, ?, ?)");
statement.setString(1, product.getName());
statement.setString(2, product.getDescription());
statement.setInt(3, product.getQuantity());
statement.setFloat(4, product.getPrice());
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
public void updateProduct(Product product) {
try {
PreparedStatement statement = connection.prepareStatement("UPDATE products SET name=?, description=?, quantity=?, price=? WHERE id=?");
statement.setString(1, product.getName());
statement.setString(2, product.getDescription());
statement.setInt(3, product.getQuantity());
statement.setFloat(4, product.getPrice());
statement.setInt(5, product.getId());
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
public void deleteProduct(int id) {
try {
PreparedStatement statement = connection.prepareStatement("DELETE FROM products WHERE id=?");
statement.setInt(1, id);
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
public Product getProductById(int id) {
try {
PreparedStatement statement = connection.prepareStatement("SELECT * FROM products WHERE id=?");
statement.setInt(1, id);
ResultSet resultSet = statement.executeQuery();
if (resultSet.next()) {
return new Product(resultSet.getInt("id"), resultSet.getString("name"), resultSet.getString("description"), resultSet.getInt("quantity"), resultSet.getFloat("price"));
}
} catch (SQLException e) {
e.printStackTrace();
}
return null;
}
public List<Product> getAllProducts() {
List<Product> products = new ArrayList<>();
try {
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM products");
while (resultSet.next()) {
products.add(new Product(resultSet.getInt("id"), resultSet.getString("name"), resultSet.getString("description"), resultSet.getInt("quantity"), resultSet.getFloat("price")));
}
} catch (SQLException e) {
e.printStackTrace();
}
return products;
}
}
```
最后,您可以在Java中使用“Inventory”类来管理仓库中的产品。示例代码如下:
```
public class Main {
public static void main(String[] args) {
Inventory inventory = new Inventory();
// Add a new product
inventory.addProduct(new Product(0, "Product 1", "Description 1", 10, 9.99f));
// Get a product by ID
Product product1 = inventory.getProductById(1);
System.out.println(product1.getName());
// Update a product
product1.setPrice(11.99f);
inventory.updateProduct(product1);
// Delete a product
inventory.deleteProduct(1);
// Get all products
List<Product> products = inventory.getAllProducts();
for (Product product : products) {
System.out.println(product.getName());
}
}
}
```
希望这可以帮助您开始编写一个简单的Java仓库管理系统,并与MySQL数据库进行交互和数据管理。
阅读全文
相关推荐
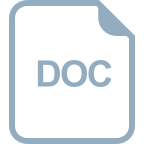
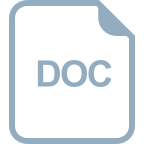
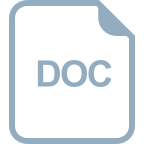
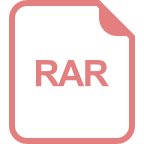
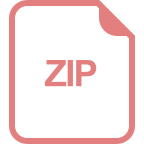
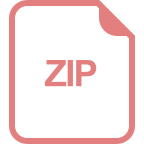
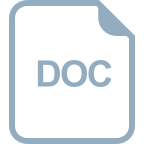
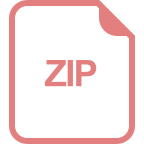
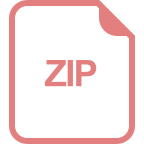
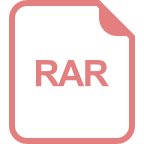
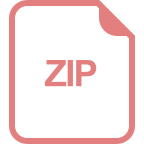
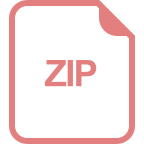
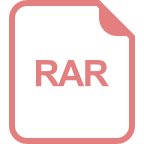
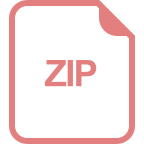
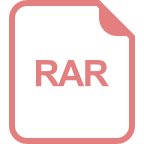
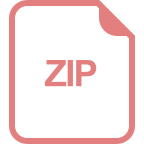
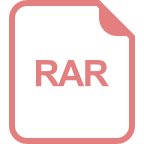
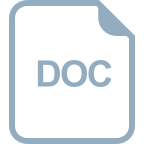
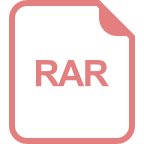