用tensorflow自定义网络层的方法步骤
时间: 2024-03-23 12:42:46 浏览: 131
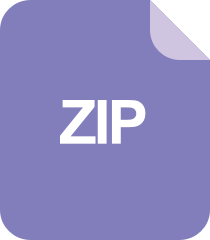
6_1_AlexNet.zip_AlexNet网络_alexnet_batch_tablelqi_watch9rp
使用 TensorFlow 自定义网络层的步骤如下:
1. 导入必要的库和模块:
```python
import tensorflow as tf
from tensorflow.keras.layers import Layer
```
2. 创建自定义层类并继承 Layer 类:
```python
class CustomLayer(Layer):
def __init__(self, arg1, arg2, **kwargs):
super(CustomLayer, self).__init__(**kwargs)
# 初始化代码
```
3. 在 `__init__` 方法中定义层需要的参数和变量:
```python
class CustomLayer(Layer):
def __init__(self, arg1, arg2, **kwargs):
super(CustomLayer, self).__init__(**kwargs)
# 初始化代码
self.arg1 = arg1
self.arg2 = arg2
self.variable1 = self.add_weight(name='variable1', shape=(1,), initializer='zeros')
```
4. 在 `build` 方法中定义层的权重:
```python
class CustomLayer(Layer):
def __init__(self, arg1, arg2, **kwargs):
super(CustomLayer, self).__init__(**kwargs)
# 初始化代码
self.arg1 = arg1
self.arg2 = arg2
def build(self, input_shape):
self.variable1 = self.add_weight(name='variable1', shape=(1,), initializer='zeros')
self.variable2 = self.add_weight(name='variable2', shape=(input_shape[-1],), initializer='random_normal', trainable=True)
```
5. 在 `call` 方法中定义层的前向传播逻辑:
```python
class CustomLayer(Layer):
def __init__(self, arg1, arg2, **kwargs):
super(CustomLayer, self).__init__(**kwargs)
# 初始化代码
self.arg1 = arg1
self.arg2 = arg2
def build(self, input_shape):
self.variable1 = self.add_weight(name='variable1', shape=(1,), initializer='zeros')
self.variable2 = self.add_weight(name='variable2', shape=(input_shape[-1],), initializer='random_normal', trainable=True)
def call(self, inputs):
output = tf.matmul(inputs, self.variable2) + self.variable1
return output
```
6. 可选:在 `get_config` 方法中定义序列化和反序列化逻辑:
```python
class CustomLayer(Layer):
def __init__(self, arg1, arg2, **kwargs):
super(CustomLayer, self).__init__(**kwargs)
# 初始化代码
self.arg1 = arg1
self.arg2 = arg2
def build(self, input_shape):
self.variable1 = self.add_weight(name='variable1', shape=(1,), initializer='zeros')
self.variable2 = self.add_weight(name='variable2', shape=(input_shape[-1],), initializer='random_normal', trainable=True)
def call(self, inputs):
output = tf.matmul(inputs, self.variable2) + self.variable1
return output
def get_config(self):
config = super(CustomLayer, self).get_config()
config.update({'arg1': self.arg1, 'arg2': self.arg2})
return config
```
7. 可选:在 `from_config` 方法中定义从配置中创建层的逻辑:
```python
class CustomLayer(Layer):
def __init__(self, arg1, arg2, **kwargs):
super(CustomLayer, self).__init__(**kwargs)
# 初始化代码
self.arg1 = arg1
self.arg2 = arg2
def build(self, input_shape):
self.variable1 = self.add_weight(name='variable1', shape=(1,), initializer='zeros')
self.variable2 = self.add_weight(name='variable2', shape=(input_shape[-1],), initializer='random_normal', trainable=True)
def call(self, inputs):
output = tf.matmul(inputs, self.variable2) + self.variable1
return output
def get_config(self):
config = super(CustomLayer, self).get_config()
config.update({'arg1': self.arg1, 'arg2': self.arg2})
return config
@classmethod
def from_config(cls, config):
return cls(**config)
```
使用自定义层时,只需将其实例化并添加到模型中即可:
```python
model = tf.keras.Sequential([
CustomLayer(arg1, arg2),
tf.keras.layers.Dense(units=10, activation='softmax'),
])
```
以上就是使用 TensorFlow 自定义网络层的步骤。
阅读全文
相关推荐
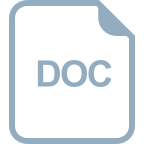
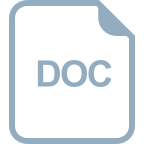


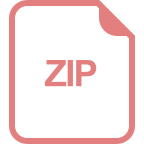
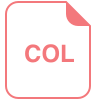
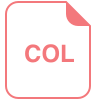

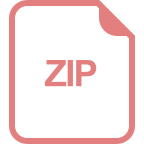
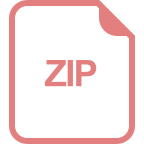
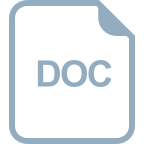
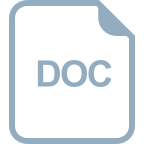
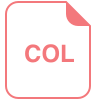
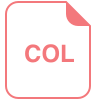
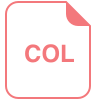

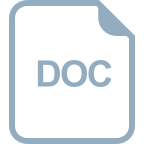
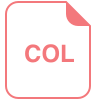