用c加加回。写一个函数,对包含n个数据的数组进行排序
时间: 2023-03-28 19:01:16 浏览: 72
好的,我可以回答这个问题。可以使用C++中的标准库函数sort()对数组进行排序,代码如下:
```c++
#include <iostream>
#include <algorithm>
using namespace std;
void sortArray(int arr[], int n) {
sort(arr, arr + n);
}
int main() {
int arr[] = {5, 2, 9, 1, 3};
int n = sizeof(arr) / sizeof(arr[]);
sortArray(arr, n);
for(int i = ; i < n; i++) {
cout << arr[i] << " ";
}
return ;
}
```
这个函数可以对任意长度的数组进行排序,只需要传入数组和数组长度即可。
相关问题
用c加加写一个快速排序
### 回答1:
用 C++ 写一个快速排序的示例代码如下: void qsort(int s[], int l, int r)
{
if (l < r)
{
int i = l, j = r;
int x = s[l];
while (i < j)
{
while(i < j && s[j] >= x)
j--;
if(i < j)
s[i++] = s[j];
while(i < j && s[i] < x)
i++;
if(i < j)
s[j--] = s[i];
}
s[i] = x;
qsort(s, l, i - 1);
qsort(s, i + 1, r);
}
}
### 回答2:
快速排序是一种常用的排序算法,在C++中可以通过递归实现。下面是一个用C++编写的快速排序的示例:
```c++
#include <iostream>
using namespace std;
// 交换数组中两个元素的值
void swap(int& a, int& b) {
int temp = a;
a = b;
b = temp;
}
// 分割数组
int partition(int arr[], int low, int high) {
int pivot = arr[high]; // 基准元素
int i = (low - 1); // 指向小于等于基准元素的索引
for (int j = low; j <= high - 1; j++) {
if (arr[j] <= pivot) {
i++;
swap(arr[i], arr[j]);
}
}
swap(arr[i + 1], arr[high]);
return (i + 1);
}
// 快速排序函数
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
int main() {
int arr[] = {10, 7, 8, 9, 1, 5};
int n = sizeof(arr) / sizeof(arr[0]);
quickSort(arr, 0, n - 1);
cout << "排序后的数组:";
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
```
这个例子中,通过`partition`函数来分割数组,选择最后一个元素作为基准(pivot)。然后使用两个指针来遍历数组,将小于等于基准的元素移到左侧,大于基准的元素移到右侧。递归调用`quickSort`函数来分别对左右两个子数组进行快速排序。最终得到排序后的数组。
### 回答3:
快速排序是一种常用的排序算法,通过递归的方式将待排序数组划分为两个子数组,使得其中一个子数组中的所有元素都小于另一个子数组中的元素。以下是用C++语言写的一个快速排序实现:
```c
#include <iostream>
using namespace std;
// 交换两个元素的位置
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
// 使用快速排序算法对数组进行排序
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pivot = arr[high]; // 选取最后一个元素作为分区点
int i = low - 1; // i记录小于分区点的元素的最右边界
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
// 将分区点放到合适的位置
swap(&arr[i + 1], &arr[high]);
//递归调用对分区点左右子数组进行排序
quickSort(arr, low, i);
quickSort(arr, i + 2, high);
}
}
int main() {
int arr[] = { 4, 2, 6, 1, 9, 3, 8, 5, 7 };
int n = sizeof(arr) / sizeof(arr[0]);
quickSort(arr, 0, n - 1);
cout << "排序后的数组:";
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
return 0;
}
```
以上代码中,快速排序算法的主要思想是通过选取一个分区点(通常为最后一个元素),将数组分成两个子数组,并保证其中一个子数组中的所有元素都小于另一个子数组中的元素。然后递归调用快速排序算法对子数组进行排序,直到每个子数组只剩下一个元素。最后将所有子数组合并起来,即可得到一个有序数组。以上代码输出的结果为`1 2 3 4 5 6 7 8 9`。
c加加如何加入一个对教师综合评分排序
要实现对教师综合评分排序,可以先定义一个结构体来存储教师的信息和评分,然后使用排序算法对结构体数组进行排序,最后输出排序结果。下面是一个简单的示例代码:
```
#include <iostream>
#include <string>
#include <algorithm>
using namespace std;
struct Teacher {
string name;
int age;
float score;
};
bool cmp(const Teacher& a, const Teacher& b) {
return a.score > b.score; // 按照评分从高到低排序
}
int main() {
const int N = 5; // 教师总数
Teacher teachers[N] = {
{"张三", 35, 4.8},
{"李四", 40, 4.5},
{"王五", 32, 4.9},
{"赵六", 45, 4.7},
{"钱七", 28, 4.6}
};
sort(teachers, teachers + N, cmp); // 排序
cout << "教师综合评分排名:" << endl;
for (int i = 0; i < N; i++) {
cout << i + 1 << ". " << teachers[i].name << ",年龄:" << teachers[i].age << ",评分:" << teachers[i].score << endl;
}
return 0;
}
```
在上面的代码中,我们先定义了一个结构体 `Teacher`,它包含了教师的姓名、年龄和评分三个属性。然后定义了一个比较函数 `cmp`,在排序时按照评分从高到低排序。最后在 `main` 函数中定义了一个结构体数组 `teachers`,存储了所有教师的信息和评分,并进行排序输出。
相关推荐
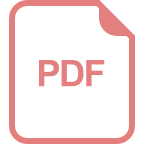










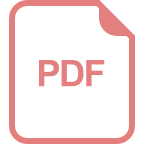