将1024*1024的图像tiff切分成为四份512*512,在训练和测试时输入网络中进行训练和测试。当四张都没有缺陷,则被认为是正常,反之则被认定为缺陷 pytorch
时间: 2024-05-03 10:17:41 浏览: 55
实现这个功能,可以使用Python中的PIL库进行图像切割,然后使用PyTorch进行训练和测试。具体的实现步骤如下:
1. 使用PIL库打开tiff图像,并获取图像的大小。
```python
from PIL import Image
img = Image.open('image.tiff')
width, height = img.size
```
2. 将图像切割成四份512*512的小图像,保存到指定目录。
```python
for i in range(0, width, 512):
for j in range(0, height, 512):
box = (i, j, i+512, j+512)
region = img.crop(box)
region.save(f'image_{i}_{j}.png')
```
3. 构建PyTorch数据集,读取图像文件并将其转换为张量。
```python
import torch
from torch.utils.data import Dataset, DataLoader
from torchvision import transforms
class ImageDataset(Dataset):
def __init__(self, image_paths):
self.image_paths = image_paths
self.transform = transforms.Compose([
transforms.Resize((512, 512)),
transforms.ToTensor()
])
def __getitem__(self, index):
image_path = self.image_paths[index]
image = Image.open(image_path)
image = self.transform(image)
return image
def __len__(self):
return len(self.image_paths)
image_paths = ['image_0_0.png', 'image_0_512.png', 'image_512_0.png', 'image_512_512.png']
dataset = ImageDataset(image_paths)
dataloader = DataLoader(dataset, batch_size=4, shuffle=True)
```
4. 定义模型、损失函数和优化器,并进行训练。
```python
import torch.nn as nn
import torch.optim as optim
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(3, 16, 3, padding=1)
self.conv2 = nn.Conv2d(16, 32, 3, padding=1)
self.fc1 = nn.Linear(32*128*128, 256)
self.fc2 = nn.Linear(256, 2)
def forward(self, x):
x = nn.functional.relu(self.conv1(x))
x = nn.functional.max_pool2d(nn.functional.relu(self.conv2(x)), 2)
x = x.view(-1, 32*128*128)
x = nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
model = Net()
criterion = nn.CrossEntropyLoss()
optimizer = optim.Adam(model.parameters(), lr=0.001)
for epoch in range(10):
running_loss = 0.0
for i, data in enumerate(dataloader, 0):
inputs = data
labels = torch.tensor([0, 0, 0, 0])
for j in range(4):
if 'defect' in image_paths[i*4+j]:
labels[j] = 1
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
running_loss += loss.item()
print(f'Epoch {epoch+1} loss: {running_loss/len(dataloader)}')
```
5. 进行测试,将四张小图像输入网络,并判断是否存在缺陷。
```python
test_image_paths = ['test_image_0_0.png', 'test_image_0_512.png', 'test_image_512_0.png', 'test_image_512_512.png']
for i, test_image_path in enumerate(test_image_paths):
test_image = Image.open(test_image_path)
test_image = transform(test_image)
test_image = test_image.unsqueeze(0)
output = model(test_image)
_, predicted = torch.max(output.data, 1)
if predicted == 1:
print(f'image_{i // 2 * 512}_{i % 2 * 512}.png has defect')
else:
print(f'image_{i // 2 * 512}_{i % 2 * 512}.png is normal')
```
这就是一个简单的图像缺陷检测系统的实现方法。当然,这只是一个基础的示例,实际应用中还需要根据具体情况进行调整和优化。
阅读全文
相关推荐
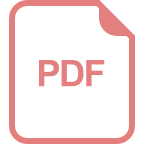
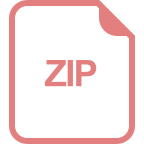
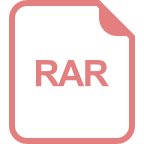
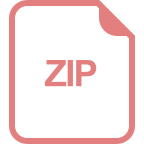
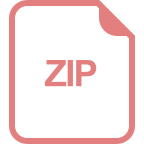
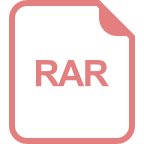
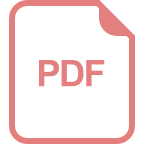
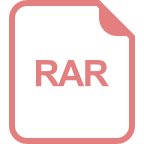
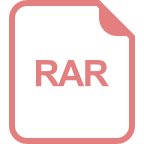
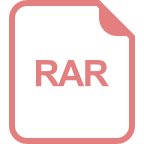
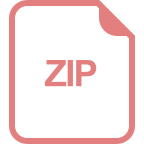
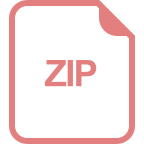
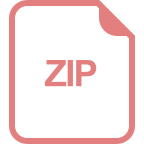
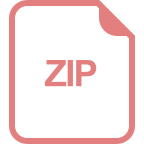
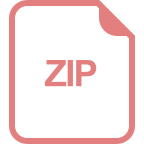