用回溯法求下列不等式的所有整数解,要求给出代码和解{3x1+4x2+2x3<=12,用C++实现
时间: 2024-12-24 08:36:36 浏览: 7
在C++中,我们可以使用回溯算法(Backtracking)结合位运算来解决这类线性不等式组的问题。由于题目要求是整数解,所以我们将范围限制在一个较小的范围内,并通过二进制搜索的方式递归地尝试每个可能的组合。以下是简单的回溯算法实现:
```cpp
#include <iostream>
#include <vector>
// 判断某个坐标是否满足不等式
bool check(const std::vector<int>& x, int target) {
int sum = 0;
for (int i = 0; i < x.size(); ++i) {
sum += x[i] * pow(2, i); // 对应于3x1, 4x2, 2x3
}
return sum <= target;
}
// 回溯函数,寻找所有整数解
std::vector<std::vector<int>> backtrack(int start, int end, const std::vector<int>& constraints) {
std::vector<std::vector<int>> solutions;
if (start > end) return solutions;
for (int i = start; i <= end; ++i) {
std::vector<int> current{x[0], x[1], x[2]};
current[0] |= i << 0; // Set x1 to the corresponding binary value of i (for 3x1)
current[1] |= (i >> 1) & 1; // Set x2 to half of i (for 4x2), shifted right by 1
current[2] |= i & 1; // Set x3 to the other half of i (for 2x3)
if (check(current, constraints)) {
solutions.push_back(current);
// Recursive call with next bit set
solutions += backtrack(i + 1, end, constraints);
}
}
return solutions;
}
int main() {
std::vector<int> constraints = {12};
std::vector<int> x = {0, 0, 0}; // Initialize x with all zeros
std::vector<std::vector<int>> results = backtrack(0, pow(2, x.size()) - 1, constraints[0]);
if (results.empty()) {
std::cout << "No integer solution found.\n";
} else {
std::cout << "Integer solutions:\n";
for (const auto& sol : results) {
std::cout << "x1=" << sol[0] << ", x2=" << sol[1] << ", x3=" << sol[2] << "\n";
}
}
return 0;
}
```
这个程序首先检查给定的目标值是否允许有非零解,然后从最低有效位开始尝试设置x的每一位,直到达到目标位数。如果当前设置满足不等式,则保存该解决方案并继续探索下一个可能的位。当所有的位都已检查完后返回结果。
注意:这里假设x1对应第一位,x2对应第二位,x3对应第三位。实际应用中可能需要调整为适应具体的变量顺序。
阅读全文
相关推荐
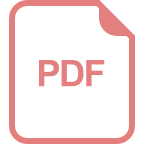
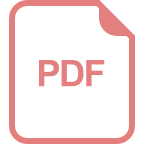






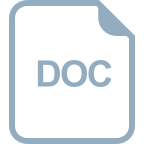




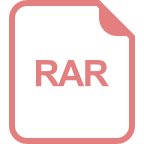
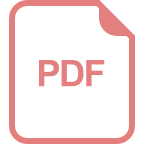
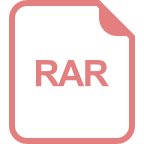
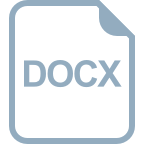