如何在Python中使用OpenCV的findContours方法进行图像轮廓的长度和宽度分析?
时间: 2024-10-27 20:03:11 浏览: 23
在Python中,使用OpenCV库(cv2模块)进行图像轮廓长度和宽度分析,首先你需要读取图片,然后运用`findContours`函数找出轮廓,接着可以遍历轮廓并计算其属性。以下是一个简单的步骤:
1. **导入必要的库**:
```python
import cv2
import numpy as np
```
2. **读取图片并转换为灰度图**:
```python
img = cv2.imread('image.jpg')
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
3. **二值化处理(如果需要)**:
```python
_, thresholded_img = cv2.threshold(gray_img, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU) # 对比度增强
```
4. **找到轮廓(contours)**:
```python
contours, _ = cv2.findContours(thresholded_img, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
5. **分析轮廓**:
- 遍历每个轮廓(contour),对每个轮廓应用`boundingRect`获取边界框,从而得到宽度和高度:
```python
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
length = max(w, h) # 轮廓的长边作为长度
width = min(w, h) # 轮廓的短边作为宽度
print(f"Contour at ({x}, {y}): Length={length}, Width={width}")
```
6. **保存结果**:
如果需要将信息可视化,你可以选择绘制轮廓到原图上。
注意:在实际操作中,`findContours`返回的是轮廓列表,`boundingRect`的结果也是一维数组,所以需要分开处理。如果你发现轮廓为空(`len(contours) == 0`),则说明没有检测到物体。
阅读全文
相关推荐
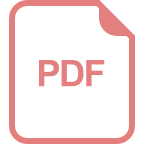
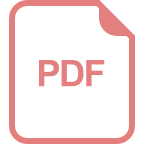
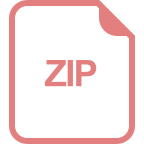
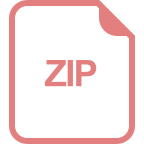
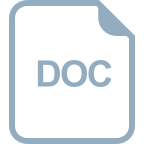
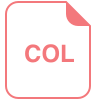
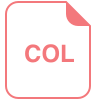
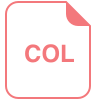
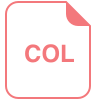
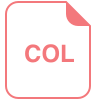
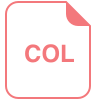
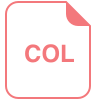
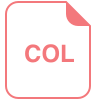
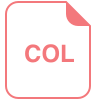
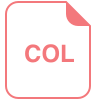
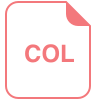
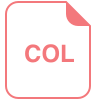

