已知长度为n的线性表L中的元素是整数,写算法求线性表中值大于a的元素个数。 要求:使用顺序表实现上述数据结构与算法。 输入样例: n=5 请输入数据元素: 5,7,9,11,12 请输入a: 6 用c语言
时间: 2024-09-13 15:07:45 浏览: 29
在C语言中,你可以使用一个循环遍历顺序表,并使用计数器记录值大于给定数字`a`的元素个数。下面是一个简单的示例算法:
```c
#include <stdio.h>
// 定义顺序表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化顺序表函数
Node* createList(int n) {
Node* head = (Node*)malloc(sizeof(Node));
head->data = 0;
head->next = NULL;
for (int i = 1; i < n; ++i) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = i + 1;
newNode->next = head->next;
head->next = newNode;
}
return head;
}
// 计算大于a的元素个数的函数
int countGreater(int a, Node* head) {
int count = 0;
Node* current = head;
while (current != NULL) {
if (current->data > a)
count++;
current = current->next;
}
return count;
}
int main() {
int n, a;
printf("请输入元素个数n: ");
scanf("%d", &n);
Node* listHead = createList(n);
printf("请输入数字a: ");
scanf("%d", &a);
int result = countGreater(a, listHead);
printf("值大于 %d 的元素个数是: %d\n", a, result);
// 清理内存
Node* temp = listHead;
while (temp != NULL) {
Node* nextTemp = temp->next;
free(temp);
temp = nextTemp;
}
return 0;
}
```
在这个程序中,首先创建了一个包含从1到n的顺序表。然后通过`countGreater`函数计算大于给定值`a`的元素数量,并在main函数中显示结果。
相关推荐
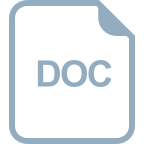
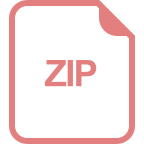
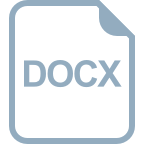














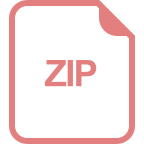
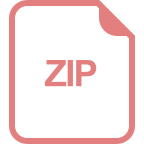