使用python,打开此文件,读取文件中第2列和第三列的数据,并提取出来不重复的行,然后将其不重复的打印出第二列和第四列的数据
时间: 2024-09-29 16:11:59 浏览: 19
在Python中,你可以使用pandas库来轻松地完成这个任务。这里是一个简单的步骤指南:
```python
import pandas as pd
# 打开文件
file_path = 'your_file_path.csv' # 替换为你的文件路径
df = pd.read_csv(file_path)
# 提取第2列和第3列的数据(假设2列为索引列)
# 我们通常不直接操作原始数据的某一列作为索引,但在假设的情况下
# df = df[['Column2', 'Column3']] # Column2和Column3是你的列名
# 或者如果你的索引是连续的整数
# df = df.iloc[:, [1, 2]]
# 去除重复行
df_unique = df.drop_duplicates(subset=['Column2']) # 'Column2'替换为你的第2列的实际列名
# 打印第二列(即之前处理过的第2列)和第四列(假设第四列为第四列,如果不是请调整下标)
print(df_unique[['Column2', 'Fourth_Column']]) # Fourth_Column替换为你实际的第四列名
# 输出结果
```
请注意,你需要确保你的列名是准确的,并且根据实际情况可能需要调整`drop_duplicates`部分的`subset`参数。
相关问题
使用python,打开此excel文件,读取文件中第2列和第三列的字符串数据并比对,并提取出来2和3不重复的行,然后将其不重复的打印出第二列和第四列的数据
在Python中,我们可以使用pandas库来处理Excel文件,实现你的需求。以下是操作步骤:
```python
import pandas as pd
# 打开Excel文件
df = pd.read_excel('your_file.xlsx') # 将 'your_file.xlsx' 替换为实际的文件路径
# 选择第2列和第3列的字符串数据(假设第一列为0,第二列为1,以此类推)
col_2 = df.iloc[:, 1] # 第二列
col_3 = df.iloc[:, 2] # 第三列
# 创建一个新的DataFrame,只包含不重复的行
unique_rows = df.drop_duplicates(subset=[col_2.name, col_3.name]) # 注意这里需要调整列名,如果原列名为'Column1'、'Column2'
# 提取第二列和第四列(如果第四列存在的话),如果不存在则跳过
if 'Column4' in df.columns:
result = unique_rows[['Column2', 'Column4']] # 把'Column2'和'Column4'替换为实际的列名
else:
result = unique_rows[['Column2']]
# 打印结果
print(result)
```
处理positionId列重复值(按照positionId去重),保留第一次出现的重复行
### 回答1:
这个问题十分合理,可以使用 Pandas 中的 drop_duplicates 函数,将 DataFrame 按照 positionId 列去重,保留第一次出现的重复行。可以这样实现:
```python
df.drop_duplicates(subset='positionId', keep='first', inplace=True)
```
其中,subset 参数指定按照哪一列去重,keep 参数指定保留哪一个重复行(这里是第一次出现的),inplace 参数指定是否修改原 DataFrame。
### 回答2:
在处理positionId列的重复值时,我们可以按照positionId去重并保留第一次出现的重复行。以下是一种处理方法:
首先,对数据进行排序,以便相同的positionId值排列在一起。
然后,创建一个空的数据选择器(selection)列表,用于存储第一次出现positionId的行索引。
接下来,遍历排序后的数据,当处理到每一行时,检查该行的positionId是否已经在选择器列表中。
如果不在选择器列表中,将该行的索引添加到选择器列表中,并继续遍历下一行。
如果已经在选择器列表中,则跳过该行,不做任何操作。
完成遍历后,选择器列表中存储的行索引即为保留的第一次出现重复行的索引。
最后,根据选择器列表的索引,重新提取原始数据中的对应行,即可得到处理后的数据,去除了positionId列的重复值,并保留了第一次出现的重复行。
以上是一种简单有效的处理方法,可以帮助我们处理positionId列的重复值。
### 回答3:
要处理positionId列的重复值,可以按照positionId这一列进行去重,只保留第一次出现的重复行。
首先,我们可以利用pandas库来处理这个问题。可以使用pandas的drop_duplicates()方法对positionId列进行去重操作。这个方法能够删除指定列中的重复行,只保留第一次出现的重复行。
具体操作如下:
1. 首先,导入pandas库。
```python
import pandas as pd
```
2. 读取包含positionId列的数据集,并将其存储为一个DataFrame对象。
```python
data = pd.read_csv('data.csv')
```
3. 使用drop_duplicates()方法对positionId列进行去重操作,并将结果重新赋值给原DataFrame对象。
```python
data = data.drop_duplicates(subset=['positionId'])
```
4. 最后,将去重后的DataFrame对象进行保存,或者进行其他需要的操作。
```python
data.to_csv('clean_data.csv', index=False)
```
通过以上步骤,我们可以实现对positionId列的去重操作,并且只保留第一次出现的重复行。最终的结果将保存为clean_data.csv文件。
相关推荐
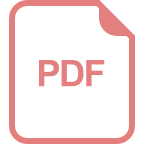
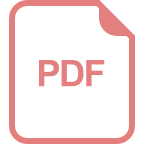
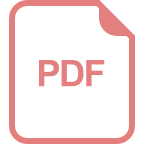











