用python代码编写一个气泡图
时间: 2024-09-10 11:23:41 浏览: 72
在Python中,我们可以使用matplotlib库来创建气泡图。气泡图是一种三维图表,其中每个点代表一个数据点,大小表示第三个变量的值,而颜色通常用于表示第四个变量。以下是一个简单的例子:
```python
import matplotlib.pyplot as plt
import numpy as np
# 创建一些随机数据
np.random.seed(0)
x = np.random.rand(50, 2) * 10
y = np.random.rand(50) * 100
s = np.random.rand(50) * 10 + 10 # 气泡大小
c = np.random.rand(50) # 颜色
# 绘制气泡图
fig, ax = plt.subplots(subplot_kw={"projection": "3d"})
ax.scatter(x[:, 0], x[:, 1], y, s=s, c=c, alpha=0.5)
# 设置坐标轴范围
ax.set_xlim([0, 10])
ax.set_ylim([0, 100])
ax.set_zlim([0, 10])
plt.show()
相关问题
Python编写一个气泡屏保
好的,下面是一个简单的气泡屏保的 Python 代码:
```python
import tkinter as tk
import random
# 定义气泡类
class Bubble:
def __init__(self, canvas, x, y):
self.canvas = canvas
self.id = canvas.create_oval(x, y, x+30, y+30, fill='white')
self.vx = random.randint(-3, 3)
self.vy = random.randint(-3, 3)
self.canvas.move(self.id, self.vx, self.vy)
def update(self):
self.canvas.move(self.id, self.vx, self.vy)
pos = self.canvas.coords(self.id)
if pos[0] <= 0 or pos[2] >= 400:
self.vx = -self.vx
if pos[1] <= 0 or pos[3] >= 300:
self.vy = -self.vy
# 创建画布
root = tk.Tk()
root.title('Bubble Screensaver')
canvas = tk.Canvas(root, width=400, height=300)
canvas.pack()
# 创建若干个气泡
bubbles = []
for i in range(20):
x = random.randint(0, 370)
y = random.randint(0, 270)
bubbles.append(Bubble(canvas, x, y))
# 更新气泡的位置
while True:
for bubble in bubbles:
bubble.update()
root.update()
tk.sleep(0.01)
```
代码中使用了 tkinter 库,它提供了创建 GUI 界面的功能。首先创建一个画布,然后定义了一个气泡类,每个气泡都有一个随机的位置和速度。在主循环中,不断更新每个气泡的位置,并通过 `tk.sleep()` 控制屏幕的刷新率。运行代码后,你就可以看到屏幕上飘荡的气泡了。
如何用Python编写一个简单的气泡图(Bubble Chart)实例?
在Python中,我们可以使用matplotlib库来创建气泡图。下面是一个简单的例子,展示了如何绘制一个二维气泡图,其中x轴代表售货机编号,y轴代表月订单量,气泡的大小表示月交易额:
```python
import matplotlib.pyplot as plt
import numpy as np
# 假设的数据
machine_ids = ['A', 'B', 'C', 'D', 'E']
transactions = [5000, 7000, 8000, 6000, 9000] # 交易额
orders = [200, 250, 300, 220, 280] # 月订单量
# 创建气泡图
fig, ax = plt.subplots()
ax.scatter(machine_ids, orders, s=transactions*100, alpha=0.5) # s参数设置气泡大小
# 添加标题和轴标签
ax.set_xlabel('售货机编号')
ax.set_ylabel('月订单量')
ax.set_title('售货机月交易额与订单量示例')
# 显示图形
plt.show()
```
在这个例子中,`scatter()`函数用于创建气泡图,`s`参数乘以100是为了将交易额转换成适中的气泡大小。运行上述代码后,你会看到一个简单的气泡图。
阅读全文
相关推荐
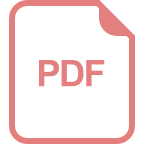
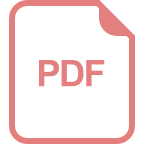
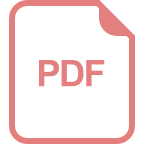
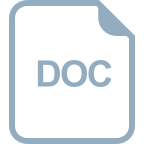
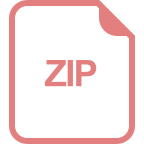
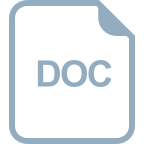


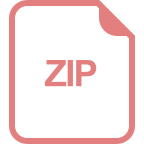
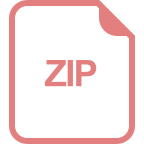
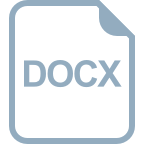
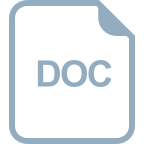




