python做泡泡龙代码
时间: 2024-10-08 17:01:08 浏览: 11
编写一个完整的Python泡泡龙游戏代码会比较长,但我可以提供一个简单的框架和步骤来帮助你开始。你需要安装`pygame`库,然后按照以下步骤构建基础功能:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置屏幕大小和标题
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Bubble Pop!")
# 定义基本颜色和字体
WHITE = (255, 255, 255)
BLUE = (0, 0, 255)
font = pygame.font.SysFont('Arial', 30)
# 创建气泡类,包含位置和颜色属性
class Bubble:
def __init__(self, x, y, color):
self.x, self.y = x, y
self.color = color
# 主函数
def main():
# 初始化游戏变量
bubbles = []
score = 0
# 游戏主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
return
# 添加新气泡
if len(bubbles) < 10:
new_bubble_pos = (random.randint(0, 790), random.randint(0, 590))
bubbles.append(Bubble(new_bubble_pos[0], new_bubble_pos[1], WHITE))
# 检查碰撞并消除气泡
for i in range(len(bubbles)):
if check_collision(i, bubbles):
remove_bubble(i, bubbles)
score += 1
# 绘制和更新
screen.fill(BLUE)
draw_bubbles(bubbles, screen)
display_score(score, screen)
pygame.display.update()
# 辅助函数
def check_collision(i, bubbles):
# 这里只是简单示例,实际游戏需要更复杂的碰撞检测
for j in range(i + 1, len(bubbles)):
if bubbles[i].y == bubbles[j].y:
return True
return False
def remove_bubble(i, bubbles):
del bubbles[i]
def draw_bubbles(bubbles, screen):
for bubble in bubbles:
pygame.draw.circle(screen, bubble.color, (bubble.x, bubble.y), 20)
def display_score(score, screen):
text = font.render(f"Score: {score}", True, WHITE)
screen.blit(text, (10, 10))
if __name__ == "__main__":
main()
```
这只是一个非常简化的版本,实际游戏中还需要添加更多细节,例如用户交互(键盘控制)、游戏结束条件以及计分系统。如果你想要深入学习,建议参考Pygame官方文档和相关教程。
相关推荐
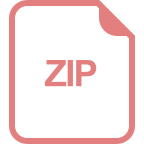
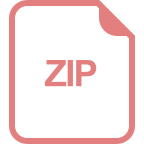
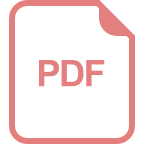














