python游戏:泡泡龙代码
时间: 2024-06-20 16:03:17 浏览: 210
泡泡龙是一款非常经典的游戏,也是很多Python初学者喜欢尝试编写的一个小游戏。下面是一个简单的Python泡泡龙游戏代码示例:
```
import pygame
from random import randrange as rnd
# 初始化pygame
pygame.init()
# 定义一些常量
WIDTH, HEIGHT = 800, 600
FPS = 50
# 创建游戏窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("Bubble Shooter")
# 加载图片
bg = pygame.image.load("background.jpg")
bubble_img = pygame.image.load("bubble.png")
# 设置字体
font = pygame.font.SysFont("Arial", 36)
# 定义颜色常量
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
# 定义泡泡类
class Bubble:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
def draw(self):
screen.blit(bubble_img, (self.x, self.y))
def move(self):
self.y -= 5
# 定义一些变量
bubbles = []
score = 0
# 添加泡泡函数
def add_bubbles():
for i in range(5):
x = rnd(100, WIDTH-100)
y = rnd(100, HEIGHT-100)
color = (rnd(0, 255), rnd(0, 255), rnd(0, 255))
bubble = Bubble(x, y, color)
bubbles.append(bubble)
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
add_bubbles()
# 绘制背景
screen.blit(bg, (0, 0))
# 绘制泡泡并移动
for bubble in bubbles:
bubble.draw()
bubble.move()
# 绘制得分
score_text = font.render("Score: " + str(score), True, WHITE)
screen.blit(score_text, (20, 20))
# 更新屏幕
pygame.display.update()
# 设置帧率
pygame.time.Clock().tick(FPS)
# 退出pygame
pygame.quit()
```
这个泡泡龙游戏代码示例使用了Python的pygame库来实现游戏窗口、图片加载、事件处理等功能,通过添加Bubble类来实现泡泡的绘制和移动,并在游戏循环中不断调用add_bubbles()函数来添加新的泡泡。同时,在游戏循环中也绘制了得分,并在按下空格键时调用add_bubbles()函数来添加新的泡泡。
阅读全文
相关推荐
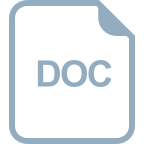
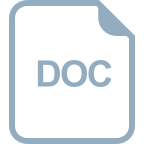
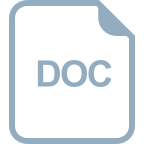
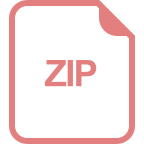


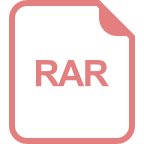
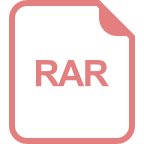
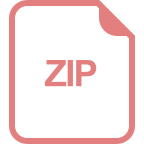
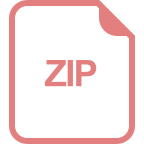
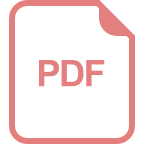
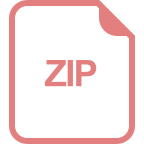
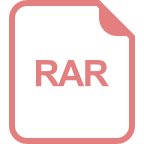
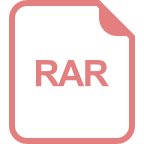
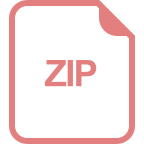
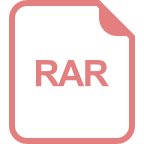
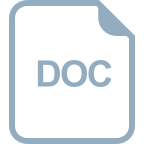
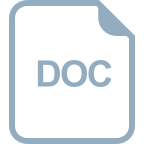
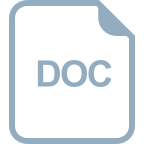
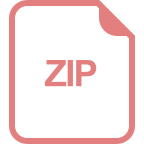