给出泡泡龙的代码用python写
时间: 2023-06-01 07:02:57 浏览: 227
以下是泡泡龙的Python代码:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 定义游戏窗口的大小
WINDOW_WIDTH = 640
WINDOW_HEIGHT = 480
# 创建游戏窗口
window = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
# 设置游戏窗口的标题
pygame.display.set_caption("Bubble Shooter")
# 定义游戏背景颜色
BG_COLOR = (255, 255, 255)
# 定义泡泡的半径
BUBBLE_RADIUS = 20
# 定义泡泡的颜色
BUBBLE_COLORS = [(255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255)]
# 定义射出的泡泡的颜色
SHOOT_BUBBLE_COLOR = (0, 0, 0)
# 定义泡泡的初始位置
BUBBLE_START_POS = (WINDOW_WIDTH // 2, WINDOW_HEIGHT - BUBBLE_RADIUS)
# 定义泡泡的速度
BUBBLE_SPEED = 5
# 定义射出泡泡的角度
SHOOT_ANGLE = 90
# 定义是否可以发射泡泡
CAN_SHOOT_BUBBLE = True
# 定义每一行泡泡的数量
BUBBLE_PER_ROW = 10
# 定义每一列泡泡的数量
BUBBLE_PER_COL = 8
# 定义泡泡之间的间距
BUBBLE_SPACING = 5
# 定义泡泡的二维数组
bubbles = []
# 初始化泡泡的二维数组
for row in range(BUBBLE_PER_COL):
bubble_row = []
for col in range(BUBBLE_PER_ROW):
bubble = {'color': random.choice(BUBBLE_COLORS), 'x': col * (BUBBLE_RADIUS * 2 + BUBBLE_SPACING) + BUBBLE_RADIUS + (WINDOW_WIDTH - (BUBBLE_RADIUS * 2 + BUBBLE_SPACING) * BUBBLE_PER_ROW) // 2, 'y': row * (BUBBLE_RADIUS * 2 + BUBBLE_SPACING) + BUBBLE_RADIUS}
bubble_row.append(bubble)
bubbles.append(bubble_row)
# 定义函数:绘制泡泡
def draw_bubble(color, x, y):
pygame.draw.circle(window, color, (x, y), BUBBLE_RADIUS)
# 定义函数:绘制射出泡泡
def draw_shoot_bubble(color, x, y):
pygame.draw.circle(window, color, (x, y), BUBBLE_RADIUS, 2)
# 定义函数:绘制泡泡数组
def draw_bubbles():
for row in bubbles:
for bubble in row:
draw_bubble(bubble['color'], bubble['x'], bubble['y'])
# 定义函数:发射泡泡
def shoot_bubble():
global CAN_SHOOT_BUBBLE
if not CAN_SHOOT_BUBBLE:
return
x = BUBBLE_START_POS[0]
y = BUBBLE_START_POS[1]
# 计算射出泡泡的速度
vel_x = BUBBLE_SPEED * math.cos(math.radians(SHOOT_ANGLE))
vel_y = -BUBBLE_SPEED * math.sin(math.radians(SHOOT_ANGLE))
while True:
x += vel_x
y += vel_y
# 判断射出泡泡是否碰到墙壁
if x - BUBBLE_RADIUS < 0 or x + BUBBLE_RADIUS > WINDOW_WIDTH:
vel_x = -vel_x
if y - BUBBLE_RADIUS < 0:
vel_y = -vel_y
if y + BUBBLE_RADIUS > WINDOW_HEIGHT:
break
# 判断射出泡泡是否碰到其他泡泡
for row in bubbles:
for bubble in row:
if bubble != None and math.sqrt((x - bubble['x']) ** 2 + (y - bubble['y']) ** 2) < BUBBLE_RADIUS * 2:
# 将射出泡泡加入泡泡数组
row_index = bubbles.index(row)
bubble_index = row.index(bubble)
bubbles[row_index][bubble_index] = {'color': SHOOT_BUBBLE_COLOR, 'x': x, 'y': y}
CAN_SHOOT_BUBBLE = True
return
# 绘制射出泡泡
window.fill(BG_COLOR)
draw_bubbles()
draw_shoot_bubble(SHOOT_BUBBLE_COLOR, x, y)
pygame.display.update()
CAN_SHOOT_BUBBLE = True
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
shoot_bubble()
# 绘制游戏界面
window.fill(BG_COLOR)
draw_bubbles()
pygame.display.update()
```
这个泡泡龙的代码是基本的,可以在此基础上进行修改和完善,比如添加碰撞检测、添加游戏分数等功能。
阅读全文
相关推荐


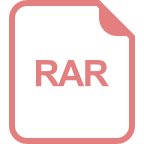
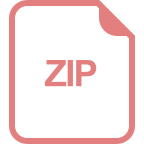
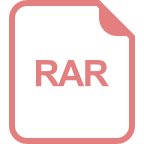
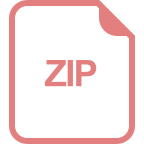
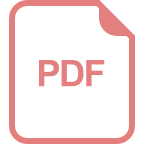
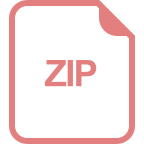
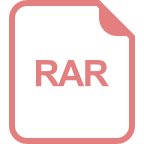
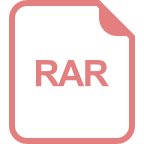
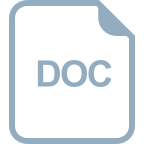