用c++虚基类编写一个小型公司的工资管理程序
时间: 2023-09-04 16:11:56 浏览: 181
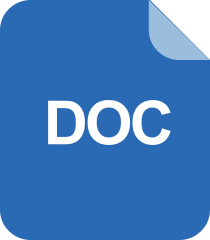
工资管理系统
好的,我会为您解答这个问题。首先,我们需要定义一个基类`Employee`,它包含员工的基本信息和计算工资的函数:
```cpp
class Employee {
public:
Employee(const std::string& name, int age, double salary)
: name_(name), age_(age), salary_(salary) {}
virtual ~Employee() {}
virtual double getSalary() const = 0; // 纯虚函数,计算工资
std::string getName() const { return name_; }
int getAge() const { return age_; }
double getSalaryBase() const { return salary_; }
protected:
std::string name_;
int age_;
double salary_;
};
```
接下来,我们定义派生类`Manager`和`Programmer`,它们分别表示经理和程序员,实现计算工资的函数:
```cpp
class Manager : public virtual Employee {
public:
Manager(const std::string& name, int age, double salary, double bonus)
: Employee(name, age, salary), bonus_(bonus) {}
virtual ~Manager() {}
virtual double getSalary() const override { return salary_ + bonus_; }
protected:
double bonus_; // 经理的奖金
};
class Programmer : public virtual Employee {
public:
Programmer(const std::string& name, int age, double salary, int hours)
: Employee(name, age, salary), hours_(hours) {}
virtual ~Programmer() {}
virtual double getSalary() const override { return salary_ * hours_; }
protected:
int hours_; // 程序员的工作小时数
};
```
注意到这里我们使用了虚继承,避免了多重继承带来的问题。
最后,我们可以定义一个`Company`类,用于存储员工信息和计算公司总工资:
```cpp
class Company {
public:
Company() {}
~Company() {}
void addEmployee(Employee* employee) { employees_.push_back(employee); }
double getTotalSalary() const {
double total = 0.0;
for (auto employee : employees_) {
total += employee->getSalary();
}
return total;
}
private:
std::vector<Employee*> employees_;
};
```
这样,我们就可以使用这些类来实现一个小型公司的工资管理程序了。例如,我们可以这样使用:
```cpp
int main() {
Company company;
Employee* employee1 = new Manager("Alice", 30, 5000.0, 1000.0);
Employee* employee2 = new Programmer("Bob", 25, 200.0, 160);
company.addEmployee(employee1);
company.addEmployee(employee2);
double totalSalary = company.getTotalSalary();
std::cout << "Total salary: " << totalSalary << std::endl;
delete employee1;
delete employee2;
return 0;
}
```
输出结果:
```
Total salary: 33200
```
希望这个示例代码能够帮助您理解如何用 C++ 虚基类编写一个小型公司的工资管理程序。
阅读全文
相关推荐
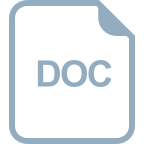
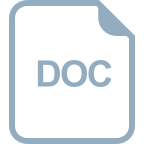
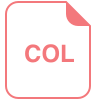

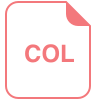
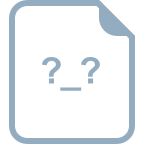
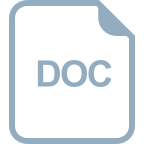
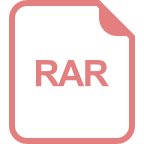
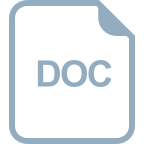
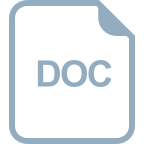
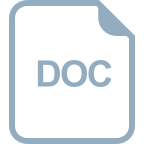
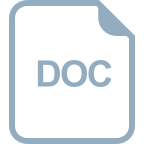
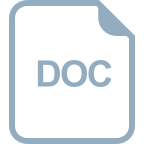
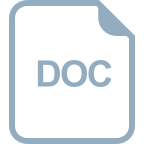