C++ DXF转NC代码
时间: 2023-12-22 17:05:17 浏览: 344
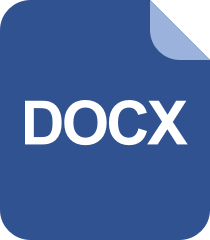
读取DXF格式文件的C++代码
DXF是一种CAD文件格式,NC代码是数控机床的控制代码,将DXF转换成NC代码可以实现将CAD图纸转换为机床加工程序。
以下是一个基于C++的DXF转NC代码的示例:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
using namespace std;
struct Point {
double x, y;
};
struct Line {
Point start, end;
};
vector<Line> parseDXF(string filename) {
vector<Line> lines;
ifstream fin(filename);
string line;
while (getline(fin, line)) {
if (line == "LINE") {
getline(fin, line);
Point start, end;
while (line != " 8") {
if (line == " 10") {
fin >> start.x;
} else if (line == " 20") {
fin >> start.y;
} else if (line == " 11") {
fin >> end.x;
} else if (line == " 21") {
fin >> end.y;
}
getline(fin, line);
}
lines.push_back({start, end});
}
}
fin.close();
return lines;
}
string toNC(Line line) {
string nc = "G01 ";
nc += "X" + to_string(line.start.x) + " ";
nc += "Y" + to_string(line.start.y) + " ";
nc += "Z0.0 ";
nc += "F500.0\n";
nc += "G01 ";
nc += "X" + to_string(line.end.x) + " ";
nc += "Y" + to_string(line.end.y) + " ";
nc += "Z0.0 ";
nc += "F500.0\n";
return nc;
}
int main() {
string dxf_filename = "example.dxf";
string nc_filename = "example.nc";
vector<Line> lines = parseDXF(dxf_filename);
ofstream fout(nc_filename);
for (Line line : lines) {
fout << toNC(line);
}
fout.close();
return 0;
}
```
以上代码实现了从DXF文件中解析出线段数据,然后将每个线段转换为NC代码字符串,最后将所有NC代码写入到文件中。这个示例代码可能还需要根据具体的DXF文件格式和NC代码格式进行调整。
阅读全文
相关推荐
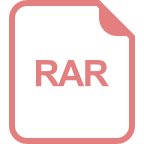
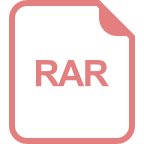
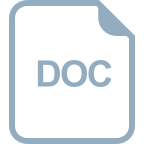
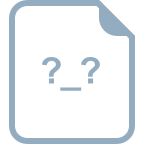
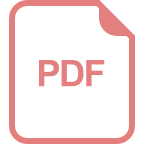
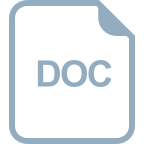
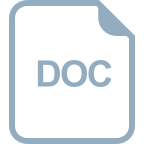
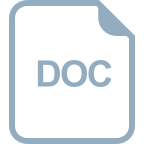
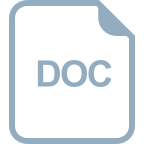
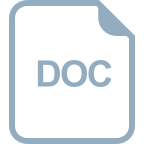
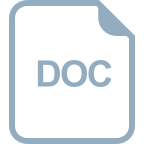

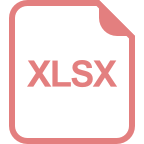
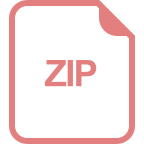
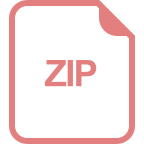
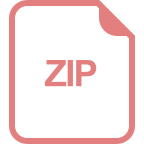
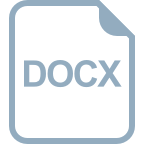