return str.replace(’openai’,’csdn’)
时间: 2023-11-06 22:07:15 浏览: 31
这是一个 Python 代码,它使用 `replace()` 方法将字符串 `str` 中的所有 `openai` 替换为 `csdn`,并返回替换后的字符串。
示例:
```python
str = "我喜欢用openai开发ChatBot。"
new_str = str.replace('openai', 'csdn')
print(new_str)
```
输出:
```
我喜欢用csdn开发ChatBot。
```
相关问题
str_replace函数用法
str_replace函数是一个字符串替换函数,在C语言中可以使用该函数来替换字符串中的指定内容。它的基本用法如下:
```c
#include <string.h>
char* str_replace(char* source, const char* find, const char* replace);
```
该函数接受三个参数:源字符串source,要查找的字符串find,以及要替换成的字符串replace。函数会在源字符串中查找所有与find相匹配的子串,并将其替换为replace。
函数返回一个指向修改后的源字符串的指针。注意,源字符串会被修改,因此调用该函数后,源字符串的内容会发生改变。
下面是一个示例代码,演示了如何使用str_replace函数:
```c
#include <stdio.h>
#include <string.h>
int main() {
char source[100] = "Hello, world!";
const char* find = "world";
const char* replace = "CSDN";
char* result = str_replace(source, find, replace);
printf("替换前的字符串: %s\n", source);
printf("替换后的字符串: %s\n", result);
return 0;
}
```
运行上述示例代码,输出结果如下:
```
替换前的字符串: Hello, world!
替换后的字符串: Hello, CSDN!
```
这样就完成了将源字符串中的"world"替换为"CSDN"的操作。注意,函数只会替换所有匹配到的子串,如果没有匹配到则不会进行替换。此外,str_replace函数是区分大小写的。如果需要进行大小写不敏感的替换,可以使用其他函数或自定义实现。
js如何使用replace
在JavaScript中,replace()是一个字符串方法,用于替换字符串中的指定内容。它接受两个参数:要替换的内容和替换后的内容。下面是使用replace()方法的几种常见方式:
1. 替换单个匹配项:
```javascript
let str = "Hello, World!";
let newStr = str.replace("World", "CSDN");
console.log(newStr); // 输出:Hello, CSDN!
```
2. 替换所有匹配项:
```javascript
let str = "Hello, World! Hello, CSDN!";
let newStr = str.replace(/Hello/g, "Hi");
console.log(newStr); // 输出:Hi, World! Hi, CSDN!
```
3. 使用正则表达式进行替换:
```javascript
let str = "Hello, World!";
let newStr = str.replace(/o/gi, "a");
console.log(newStr); // 输出:Hella, Warld!
```
4. 使用函数进行替换:
```javascript
let str = "Hello, World!";
let newStr = str.replace(/o/gi, function(match) {
return match.toUpperCase();
});
console.log(newStr); // 输出:HellO, WOrld!
```
相关推荐
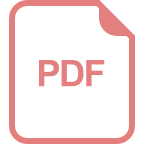












