定义一个结构体变量(包括年、月、日)。计算该日在本年中是第几天?注意闰年问题。输出格式要求: "\n%d月%d日是%d年的第%d天。 程序的运行示例如下: 请输入日期(年,月,日) 1990,2, 14 2月14日是1990年的第45天。
时间: 2023-12-03 21:06:11 浏览: 66
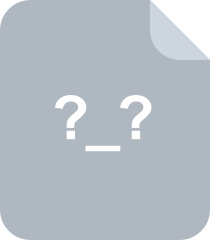
1.定义一个结构体变量(包括年、月、日)。计算该日在本年中是第几天,注意闰年的问题。
下面是一个 C 语言的示例程序,可以实现上述功能:
```c
#include <stdio.h>
struct Date {
int year;
int month;
int day;
};
int daysInMonth(int year, int month) {
static const int days[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
if (month == 2 && (year % 4 == 0 && year % 100 != 0 || year % 400 == 0)) {
return 29; // Leap year
} else {
return days[month - 1];
}
}
int daysInYear(int year) {
return daysInMonth(year, 12) + 335;
}
int daysToDate(struct Date date) {
int days = 0;
for (int month = 1; month < date.month; month++) {
days += daysInMonth(date.year, month);
}
days += date.day;
if (date.month > 2 && daysInMonth(date.year, 2) == 29) {
days++; // Leap day
}
return days;
}
int main() {
struct Date date;
printf("请输入日期(年,月,日): ");
scanf("%d,%d,%d", &date.year, &date.month, &date.day);
int days = daysToDate(date);
printf("%d月%d日是%d年的第%d天。\n", date.month, date.day, date.year, days);
return 0;
}
```
该程序使用结构体 `Date` 表示一个日期,其中包含年、月、日三个字段。
函数 `daysInMonth(year, month)` 返回指定年份和月份的天数,考虑了闰年的情况。如果指定的年份是闰年,且要求的月份是 2 月,则返回 29 天;否则返回一个数组中对应的天数。
函数 `daysInYear(year)` 返回指定年份的总天数,包括 12 月份的天数和前面 11 个月的天数。
函数 `daysToDate(date)` 返回指定日期在该年中的天数,计算方法是先累加前面几个月的天数,再加上当前月的天数。如果当前月是 2 月,且该年是闰年,则还要加上一个闰日。
在 `main()` 函数中,程序首先提示用户输入日期,然后调用 `scanf()` 函数读入年、月、日三个字段的值。接着调用 `daysToDate()` 函数计算该日期在该年中的天数,并输出结果。输出格式符合题目要求。
阅读全文
相关推荐
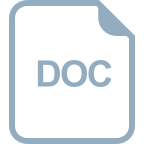















