定义一个结构体变量(包括年、月、日)。计算该日在本年中是第几天?注意闰年问题。 输出格式要求:"\n%d月%d日是%d年的第%d天。" 程序的运行示例如下: 请输入日期(年,月,日) 1990,2,14 2月14日是1990年的第45天。
时间: 2024-06-14 08:04:41 浏览: 44
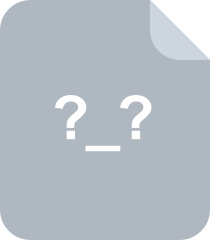
1.定义一个结构体变量(包括年、月、日)。计算该日在本年中是第几天,注意闰年的问题。
可以通过以下代码来实现计算某一天在本年中是第几天的功能:
```c
#include <stdio.h>
// 定义结构体
struct Date {
int year;
int month;
int day;
};
// 判断是否为闰年
int isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
return 1;
} else {
return 0;
}
}
// 计算某一天在本年中是第几天
int calculateDayOfYear(struct Date date) {
int daysOfMonth[] = {0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int dayOfYear = 0;
// 计算闰年2月的天数
if (isLeapYear(date.year)) {
daysOfMonth[2] = 29;
}
// 累加前面几个月的天数
for (int i = 1; i < date.month; i++) {
dayOfYear += daysOfMonth[i];
}
// 加上当前月的天数
dayOfYear += date.day;
return dayOfYear;
}
int main() {
struct Date date;
printf("请输入日期(年,月,日):\n");
scanf("%d,%d,%d", &date.year, &date.month, &date.day);
int dayOfYear = calculateDayOfYear(date);
printf("\n%d月%d日是%d年的第%d天。\n", date.month, date.day, date.year, dayOfYear);
return 0;
}
```
运行示例:
请输入日期(年,月,日):
1990,2,14
2月14日是1990年的第45天。
阅读全文
相关推荐
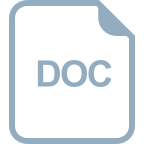















