ffmpeg 解码实时视频流
时间: 2023-07-28 17:09:29 浏览: 125
您好!对于实时视频流的解码,可以使用FFmpeg库来实现。以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <unistd.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/imgutils.h>
#include <libswscale/swscale.h>
int main(int argc, char *argv[]) {
AVFormatContext *formatContext = NULL;
AVCodecContext *codecContext = NULL;
AVCodec *codec = NULL;
AVPacket packet;
AVFrame *frame = NULL;
struct SwsContext *swsContext = NULL;
int videoStreamIndex = -1;
int ret;
if (argc < 2) {
printf("Usage: %s <input_stream>\n", argv[0]);
return -1;
}
av_register_all();
// 打开视频流
if (avformat_open_input(&formatContext, argv[1], NULL, NULL) != 0) {
printf("Error opening the input stream.\n");
return -1;
}
// 获取视频流信息
if (avformat_find_stream_info(formatContext, NULL) < 0) {
printf("Error finding the stream information.\n");
return -1;
}
// 查找视频流
for (int i = 0; i < formatContext->nb_streams; i++) {
if (formatContext->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
videoStreamIndex = i;
break;
}
}
if (videoStreamIndex == -1) {
printf("No video stream found.\n");
return -1;
}
// 获取视频解码器参数
codecContext = avcodec_alloc_context3(NULL);
if (codecContext == NULL) {
printf("Failed to allocate codec context.\n");
return -1;
}
avcodec_parameters_to_context(codecContext, formatContext->streams[videoStreamIndex]->codecpar);
// 查找解码器
codec = avcodec_find_decoder(codecContext->codec_id);
if (codec == NULL) {
printf("Unsupported codec.\n");
return -1;
}
// 打开解码器
if (avcodec_open2(codecContext, codec, NULL) < 0) {
printf("Failed to open codec.\n");
return -1;
}
// 创建帧
frame = av_frame_alloc();
if (frame == NULL) {
printf("Failed to allocate frame.\n");
return -1;
}
// 初始化数据包
av_init_packet(&packet);
// 读取视频流
while (av_read_frame(formatContext, &packet) >= 0) {
if (packet.stream_index == videoStreamIndex) {
// 解码视频帧
ret = avcodec_send_packet(codecContext, &packet);
if (ret < 0) {
printf("Error sending a packet for decoding.\n");
break;
}
while (ret >= 0) {
ret = avcodec_receive_frame(codecContext, frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF)
break;
else if (ret < 0) {
printf("Error during decoding.\n");
break;
}
// 进行图像处理,这里可以根据需要进行各种操作,如保存图像、显示图像等
// 在这个例子中,我们只是简单地将解码后的帧保存为PPM格式的图像文件
// 转换图像格式
swsContext = sws_getCachedContext(swsContext,
frame->width,
frame->height,
codecContext->pix_fmt,
frame->width,
frame->height,
AV_PIX_FMT_RGB24,
SWS_BILINEAR,
NULL, NULL, NULL);
if (!swsContext) {
printf("Failed to allocate SwsContext.\n");
return -1;
}
AVFrame *rgbFrame = av_frame_alloc();
if (!rgbFrame) {
printf("Failed to allocate RGB frame.\n");
return -1;
}
av_image_alloc(rgbFrame->data, rgbFrame->linesize, frame->width, frame->height, AV_PIX_FMT_RGB24, 1);
sws_scale(swsContext, (const uint8_t *const *) frame->data, frame->linesize, 0, frame->height,
rgbFrame->data, rgbFrame->linesize);
// 保存图像
char filename[32];
sprintf(filename, "frame-%d.ppm", codecContext->frame_number);
FILE *file = fopen(filename, "wb");
if (file) {
fprintf(file, "P6\n%d %d\n255\n", frame->width, frame->height);
fwrite(rgbFrame->data[0], 1, frame->width * frame->height * 3, file);
fclose(file);
}
av_frame_free(&rgbFrame);
}
}
av_packet_unref(&packet);
}
// 清理资源
av_frame_free(&frame);
avcodec_close(codecContext);
avformat_close_input(&formatContext);
return 0;
}
```
上述代码使用FFmpeg库对输入视频流进行解码,并将解码后的帧保存为PPM格式的图像文件。您可以根据需要进行修改和扩展以满足您的实际需求。希望对您有所帮助!如果有任何疑问,请随时提问。
相关推荐
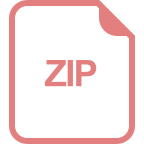














