quarkus 下载图片打包成zip文件让web如何接收压缩包地址
时间: 2023-12-25 20:06:59 浏览: 146
在 Quarkus 中,可以使用 REST API 来实现文件下载和压缩。以下是一个简单的例子:
1. 创建一个 REST 资源类来处理文件下载和压缩:
```
@Path("/download")
public class FileResource {
@GET
@Path("/image/{fileName}")
@Produces(MediaType.APPLICATION_OCTET_STREAM)
public Response downloadImage(@PathParam("fileName") String fileName) {
// 从服务器获取要下载的图片
File file = new File("/path/to/images/" + fileName);
ResponseBuilder response = Response.ok((Object) file);
response.header("Content-Disposition", "attachment; filename=" + fileName);
return response.build();
}
@GET
@Path("/zip")
@Produces("application/zip")
public Response downloadZip() {
// 创建要下载的 ZIP 文件
File zipFile = createZipFile();
ResponseBuilder response = Response.ok((Object) zipFile);
response.header("Content-Disposition", "attachment; filename=images.zip");
return response.build();
}
private File createZipFile() {
// 将多个文件打包成 ZIP 文件
// ...
}
}
```
2. 在 Quarkus 应用程序中注册资源类:
```
@ApplicationScoped
public class MyApplication extends Application {
@Override
public Set<Class<?>> getClasses() {
Set<Class<?>> classes = new HashSet<>();
classes.add(FileResource.class);
return classes;
}
}
```
3. 在 Web 界面中使用 AJAX 请求来下载和解压缩 ZIP 文件:
```
$.ajax({
url: '/download/zip',
type: 'GET',
success: function(data) {
// 将 ZIP 文件解压缩并显示其中的图片
JSZip.loadAsync(data).then(function(zip) {
zip.forEach(function (relativePath, file) {
if (!file.dir) {
var img = document.createElement('img');
img.src = URL.createObjectURL(file._data.compressedContent);
document.body.appendChild(img);
}
});
});
}
});
```
这样,当用户在 Web 界面中点击下载按钮时,将会触发 AJAX 请求来下载 ZIP 文件,并将其中的图片解压缩并显示在页面上。
阅读全文
相关推荐
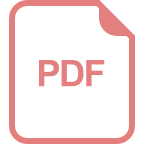
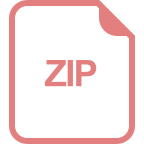
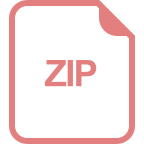
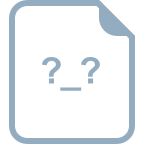
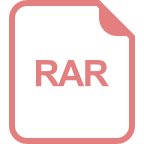
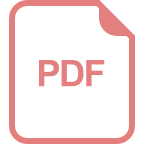
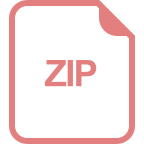
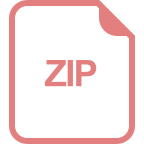
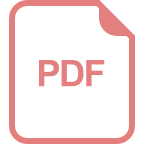
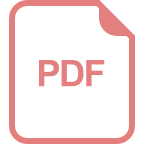
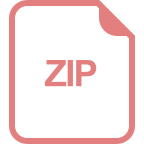
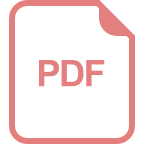