C语言 结构体数组 转josn
时间: 2023-08-26 21:06:20 浏览: 105
要将C语言中的结构体数组转换为JSON格式,你可以使用第三方库,如 cJSON 或 Jansson。
以下是使用 cJSON 库的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <cJSON.h>
typedef struct {
char name[50];
int age;
double salary;
} Employee;
int main() {
// 创建一个包含多个 Employee 结构体的数组
Employee employees[3];
strcpy(employees[0].name, "John Doe");
employees[0].age = 30;
employees[0].salary = 5000.0;
strcpy(employees[1].name, "Jane Smith");
employees[1].age = 35;
employees[1].salary = 6000.0;
strcpy(employees[2].name, "Mike Johnson");
employees[2].age = 40;
employees[2].salary = 7000.0;
// 创建一个 JSON 数组
cJSON *root = cJSON_CreateArray();
// 遍历结构体数组,将每个结构体转换为 JSON 对象,并添加到 JSON 数组中
for (int i = 0; i < 3; i++) {
cJSON *employeeObj = cJSON_CreateObject();
cJSON_AddStringToObject(employeeObj, "name", employees[i].name);
cJSON_AddNumberToObject(employeeObj, "age", employees[i].age);
cJSON_AddNumberToObject(employeeObj, "salary", employees[i].salary);
cJSON_AddItemToArray(root, employeeObj);
}
// 将 JSON 数组转换为字符串
char *jsonStr = cJSON_Print(root);
// 打印 JSON 字符串
printf("%s\n", jsonStr);
// 释放内存
cJSON_Delete(root);
free(jsonStr);
return 0;
}
```
注意,上述示例代码需要先安装 cJSON 库,并在编译时链接该库。编译命令如下:
```bash
gcc example.c -o example -lcjson
```
执行编译后的可执行文件将输出转换后的JSON字符串。
你可以根据自己的需求修改结构体数组和对应的JSON字段。
阅读全文
相关推荐
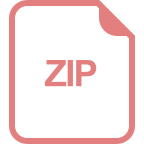
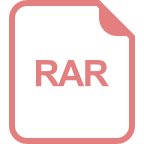
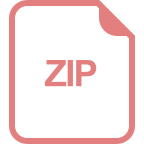











