Java创建text文件路径并保存指定服务器
时间: 2024-09-24 21:19:17 浏览: 25
在Java中,创建文本文件并保存到远程服务器通常涉及到两个步骤:首先构造文件路径,然后通过网络IO操作将数据写入文件。这里是一个基本示例:
```java
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
public class FileSavingExample {
private static final String REMOTE_SERVER_URL = "http://your-server-url.com/path/to/save"; // 替换为你服务器的实际地址
public static void main(String[] args) {
try {
// 构造文件名
String fileName = "example.txt";
// 文件内容
String content = "This is the content to be saved on the server.";
// 创建文件路径
URL url = new URL(REMOTE_SERVER_URL + "/" + fileName);
// 创建HttpURLConnection实例
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "text/plain"); // 设置请求内容类型
// 将数据写入连接的输出流
BufferedWriter writer = new BufferedWriter(new FileWriter(connection.getOutputStream()));
writer.write(content);
writer.close();
// 检查HTTP响应状态码
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
System.out.println("Data saved successfully at " + url);
} else {
System.out.println("Failed to save data. Server responded with code: " + responseCode);
}
} catch (IOException e) {
e.printStackTrace();
System.out.println("An error occurred while saving the file.");
}
}
}
```
相关推荐
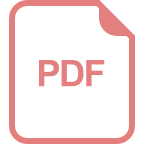
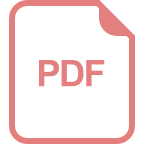
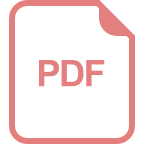














