python明文加密
时间: 2023-08-16 16:15:10 浏览: 62
在Python中,可以使用多种方式对明文进行加密。其中,常见的加密算法包括:
1. Caesar密码:Caesar密码是一种简单的替换密码,通过将明文中的每个字符按照一定的偏移量进行替换来实现加密。可以使用循环和ASCII码来实现该算法。
```python
def caesar_encrypt(plaintext, key):
ciphertext = ''
for char in plaintext:
if char.isalpha():
ascii_offset = ord('a') if char.islower() else ord('A')
encrypted_char = chr((ord(char) - ascii_offset + key) % 26 + ascii_offset)
ciphertext += encrypted_char
else:
ciphertext += char
return ciphertext
plaintext = 'Hello, World!'
key = 3
encrypted_text = caesar_encrypt(plaintext, key)
print(encrypted_text) # Output: Khoor, Zruog!
```
2. AES加密:AES(Advanced Encryption Standard)是一种对称加密算法,可以用于保护敏感数据。在Python中,可以使用`pycryptodome`库来实现AES加密。
```python
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad
def aes_encrypt(plaintext, key):
cipher = AES.new(key.encode(), AES.MODE_ECB)
padded_plaintext = pad(plaintext.encode(), AES.block_size)
ciphertext = cipher.encrypt(padded_plaintext)
return ciphertext.hex()
plaintext = 'Hello, World!'
key = '0123456789abcdef' # 16字节的AES密钥
encrypted_text = aes_encrypt(plaintext, key)
print(encrypted_text) # Output: 73f3857d5f0b2ac7d3117a8e3e152f4a
```
这只是两种常见的加密算法示例,实际上还有很多其他的加密算法可供选择,如DES、RSA等。请根据具体需求选择适合的加密算法。
相关推荐
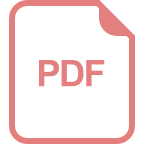
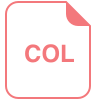
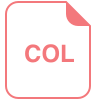
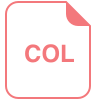
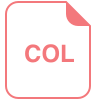
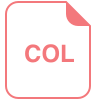









