c++用链表类实现堆栈
时间: 2023-08-15 16:24:01 浏览: 123
以下是使用链表类实现堆栈的C++代码:
```c++
#include <iostream>
using namespace std;
class Node {
public:
int data;
Node* next;
};
class Stack {
private:
Node* top;
public:
Stack() {
top = nullptr;
}
void push(int val) {
Node* newNode = new Node();
newNode->data = val;
newNode->next = top;
top = newNode;
}
bool isEmpty() {
return top == nullptr;
}
int peek() {
if (!isEmpty()) {
return top->data;
} else {
cout << "Stack is empty!" << endl;
return -1;
}
}
void pop() {
if (!isEmpty()) {
Node* temp = top;
top = top->next;
delete temp;
} else {
cout << "Stack is empty!" << endl;
}
}
};
int main() {
Stack s;
s.push(5);
s.push(10);
s.push(15);
cout << s.peek() << endl;
s.pop();
cout << s.peek() << endl;
s.pop();
cout << s.peek() << endl;
s.pop();
cout << s.peek() << endl;
return 0;
}
```
在这个代码中,我们定义了两个类:`Node`和`Stack`。`Node`类表示堆栈中的一个节点,`Stack`类表示堆栈本身。在`Stack`类中,我们使用了链表来实现堆栈。具体地,`top`指针指向堆栈的顶部。当我们向堆栈中添加一个元素时,我们创建一个新的节点,将其指向当前的`top`节点,然后将`top`指针指向新的节点。当我们从堆栈中删除一个元素时,我们删除顶部节点,并将`top`指针指向下一个节点。
在`main`函数中,我们创建了一个堆栈对象`s`,并向其中添加了三个元素。然后,我们使用`peek`函数查看堆栈的顶部元素,并使用`pop`函数删除堆栈的顶部元素。最后,我们使用`peek`函数再次查看堆栈的顶部元素。
阅读全文
相关推荐
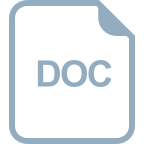
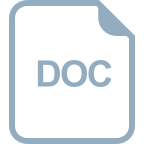
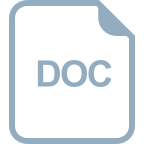
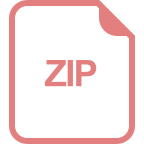
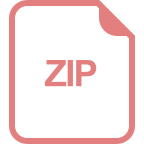
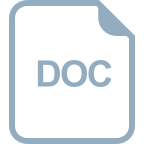
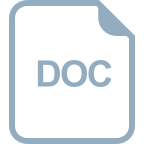
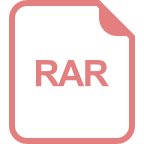
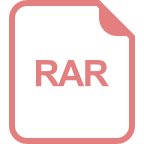
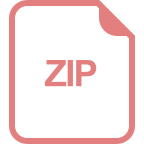
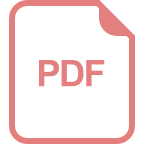
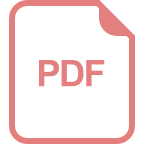
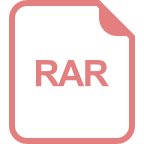
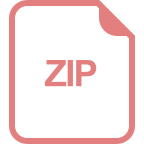
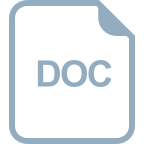
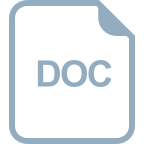
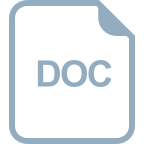
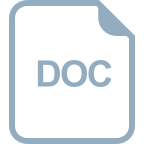
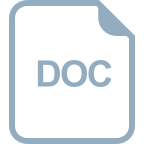