用element-ui的表格组件生成一个可上下拖拽的组件
时间: 2023-12-27 09:03:09 浏览: 30
你可以使用 Element UI 的 `<el-table>` 组件结合拖拽库,实现一个可以上下拖拽的表格组件。以下是一个示例代码:
```vue
<template>
<div>
<el-table
:data="tableData"
:row-key="getRowKey"
ref="dragTable"
:row-class-name="getRowClassName"
>
<el-table-column label="序号" width="100">
<template slot-scope="{ row, $index }">
<span>{{ $index + 1 }}</span>
</template>
</el-table-column>
<el-table-column label="姓名">
<template slot-scope="{ row }">
<span>{{ row.name }}</span>
</template>
</el-table-column>
<el-table-column label="年龄">
<template slot-scope="{ row }">
<span>{{ row.age }}</span>
</template>
</el-table-column>
</el-table>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [
{ name: '张三', age: 20 },
{ name: '李四', age: 25 },
{ name: '王五', age: 30 },
{ name: '赵六', age: 35 },
],
};
},
methods: {
getRowKey(row) {
return row.name; // 根据实际情况返回唯一的键值
},
getRowClassName(row, index) {
return `row-${index}`;
},
handleDragEnd(event) {
const { newIndex, oldIndex } = event;
const draggedRow = this.tableData.splice(oldIndex, 1)[0];
this.tableData.splice(newIndex, 0, draggedRow);
// 后续可以在此处将新的顺序保存到数据库或进行其他操作
},
},
mounted() {
const dragTable = this.$refs.dragTable.$el.querySelector('.el-table__body-wrapper');
const sortable = new Sortable(dragTable, {
animation: 150,
handle: '.el-table__row',
ghostClass: 'dragging',
});
sortable.on('end', this.handleDragEnd);
},
};
</script>
<style scoped>
.dragging {
opacity: 0.5;
}
</style>
```
在上面的示例中,我们使用了 `<el-table>` 组件来展示表格数据,通过设置 `:row-key` 属性来指定行的唯一键值。然后,我们使用了一个自定义的样式类名 `row-${index}` 来为每一行添加样式,以便在拖拽时添加视觉效果。我们还使用了 Sortable.js 库来实现拖拽功能,并监听了拖拽结束的事件来更新表格数据的顺序。
注意:在使用此示例代码之前,请确保已安装 Sortable.js 库,并在 Vue 组件中进行引入。
希望对你有所帮助!
相关推荐
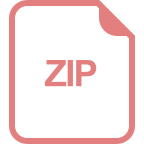
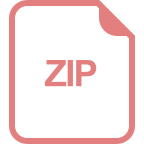
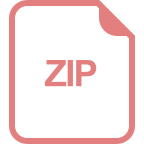














