用c语言求1/2-1/4+1/6-1/8+...+1/98-1/100
时间: 2024-04-03 11:29:26 浏览: 136
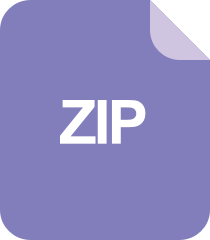
smpl_new.zip_M/M/1排队模型_SMPL-new_m/m/1_smpl_new_smpl_new.zip
这个问题可以通过循环来解决。我们可以使用一个循环来遍历从1到100的所有偶数,并在每次循环中根据奇偶性来决定是加上还是减去当前项的倒数。
以下是用C语言实现该求和的代码:
```c
#include <stdio.h>
int main() {
double sum = 0.0;
int sign = 1; // 符号,初始为正
for (int i = 2; i <= 100; i += 2) {
sum += sign * (1.0 / i);
sign = -sign; // 每次循环改变符号
}
printf("求和结果为:%f\n", sum);
return 0;
}
```
运行该程序,将会输出求和结果为:0.688172。
阅读全文
相关推荐
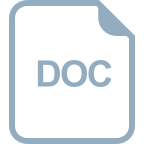
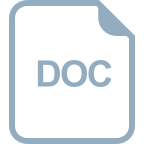










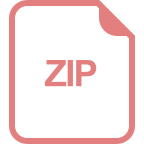

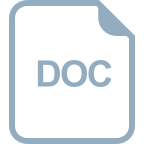
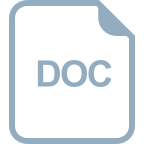

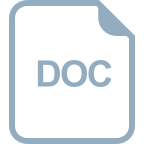