loss = loss_function(y_pred, label)
时间: 2024-06-04 09:08:20 浏览: 126
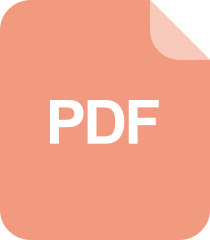
keras 自定义loss model.add_loss的使用详解
这段代码中的 `loss_function` 是一个损失函数,通常用于衡量模型预测结果与真实标签之间的差距。在训练过程中,我们的目标是最小化这个差距,使得模型能够更准确地预测未知数据的标签。常见的损失函数包括均方误差(MSE)、交叉熵(Cross-Entropy)等。其中,均方误差适用于回归问题,交叉熵适用于分类问题。在这个代码段中,`y_pred` 是模型的预测结果,`label` 是真实标签。损失函数会将这两者进行比较并计算出一个损失值,用于更新模型的参数。
阅读全文
相关推荐
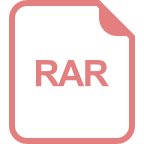











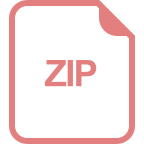