给我用Java写一个二分查询list中最大的数的下标,
时间: 2024-04-14 14:25:48 浏览: 12
当然可以!下面是用 Java 实现二分查找列表中最大数的索引的示例代码:
```java
import java.util.List;
public class BinarySearchExample {
public static int binarySearchMaxIndex(List<Integer> list) {
int left = 0;
int right = list.size() - 1;
int maxIndex = -1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (list.get(mid) > list.get(maxIndex)) {
maxIndex = mid;
}
if (list.get(mid) > list.get(mid + 1)) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return maxIndex;
}
public static void main(String[] args) {
List<Integer> list = List.of(1, 3, 5, 7, 9, 8, 6, 4, 2);
int maxIndex = binarySearchMaxIndex(list);
System.out.println("Max index: " + maxIndex);
}
}
```
在这个示例代码中,我们使用了经典的二分查找算法。首先,我们初始化左边界 `left` 为列表的起始位置,右边界 `right` 为列表的结束位置。同时,我们还定义了一个 `maxIndex` 变量,用于保存当前找到的最大数的索引。
然后,我们进入一个循环,直到左边界大于右边界。在每次循环中,我们首先计算出中间位置 `mid`,然后通过比较 `list.get(mid)` 和 `list.get(maxIndex)` 的值,更新 `maxIndex`。接着,我们根据 `list.get(mid)` 和 `list.get(mid + 1)` 的大小关系,调整左边界和右边界的位置。
最终,循环结束后,`maxIndex` 中保存的就是列表中最大数的索引。在示例的 `main` 方法中,我们给定了一个示例列表,并调用 `binarySearchMaxIndex` 方法来获取最大数的索引,并将结果打印输出。
希望这个示例代码对你有帮助!如果有任何问题,请随时问我。
相关推荐
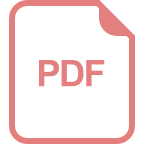
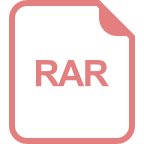
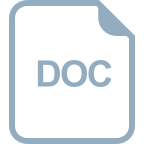














