简单写一个VGG神经网络代码
时间: 2023-07-22 10:28:03 浏览: 52
好的,以下是一个简单的VGG神经网络代码,包含了VGG16和VGG19两个模型的实现:
```python
import tensorflow as tf
def conv_block(inputs, num_filters, kernel_size, strides, padding):
x = tf.keras.layers.Conv2D(num_filters, kernel_size, strides=strides, padding=padding)(inputs)
x = tf.keras.layers.BatchNormalization()(x)
x = tf.keras.layers.Activation('relu')(x)
return x
def vgg16(input_shape=(224, 224, 3), num_classes=1000):
inputs = tf.keras.layers.Input(shape=input_shape)
x = conv_block(inputs, 64, 3, 1, 'same')
x = conv_block(x, 64, 3, 1, 'same')
x = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(x)
x = conv_block(x, 128, 3, 1, 'same')
x = conv_block(x, 128, 3, 1, 'same')
x = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(x)
x = conv_block(x, 256, 3, 1, 'same')
x = conv_block(x, 256, 3, 1, 'same')
x = conv_block(x, 256, 3, 1, 'same')
x = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(x)
x = conv_block(x, 512, 3, 1, 'same')
x = conv_block(x, 512, 3, 1, 'same')
x = conv_block(x, 512, 3, 1, 'same')
x = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(x)
x = conv_block(x, 512, 3, 1, 'same')
x = conv_block(x, 512, 3, 1, 'same')
x = conv_block(x, 512, 3, 1, 'same')
x = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(x)
x = tf.keras.layers.Flatten()(x)
x = tf.keras.layers.Dense(4096, activation='relu')(x)
x = tf.keras.layers.Dropout(0.5)(x)
x = tf.keras.layers.Dense(4096, activation='relu')(x)
x = tf.keras.layers.Dropout(0.5)(x)
outputs = tf.keras.layers.Dense(num_classes, activation='softmax')(x)
model = tf.keras.models.Model(inputs, outputs)
return model
def vgg19(input_shape=(224, 224, 3), num_classes=1000):
inputs = tf.keras.layers.Input(shape=input_shape)
x = conv_block(inputs, 64, 3, 1, 'same')
x = conv_block(x, 64, 3, 1, 'same')
x = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(x)
x = conv_block(x, 128, 3, 1, 'same')
x = conv_block(x, 128, 3, 1, 'same')
x = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(x)
x = conv_block(x, 256, 3, 1, 'same')
x = conv_block(x, 256, 3, 1, 'same')
x = conv_block(x, 256, 3, 1, 'same')
x = conv_block(x, 256, 3, 1, 'same')
x = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(x)
x = conv_block(x, 512, 3, 1, 'same')
x = conv_block(x, 512, 3, 1, 'same')
x = conv_block(x, 512, 3, 1, 'same')
x = conv_block(x, 512, 3, 1, 'same')
x = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(x)
x = conv_block(x, 512, 3, 1, 'same')
x = conv_block(x, 512, 3, 1, 'same')
x = conv_block(x, 512, 3, 1, 'same')
x = conv_block(x, 512, 3, 1, 'same')
x = tf.keras.layers.MaxPooling2D(pool_size=(2, 2))(x)
x = tf.keras.layers.Flatten()(x)
x = tf.keras.layers.Dense(4096, activation='relu')(x)
x = tf.keras.layers.Dropout(0.5)(x)
x = tf.keras.layers.Dense(4096, activation='relu')(x)
x = tf.keras.layers.Dropout(0.5)(x)
outputs = tf.keras.layers.Dense(num_classes, activation='softmax')(x)
model = tf.keras.models.Model(inputs, outputs)
return model
```
以上代码中,我们定义了一个名为conv_block的函数,用于构建卷积块。然后我们按照VGG16和VGG19的结构,依次堆叠卷积块和池化层,最后接上全连接层进行分类。
可以根据实际需要,调整输入图片的大小和分类的类别数量。
相关推荐
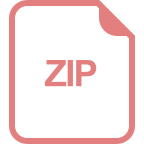
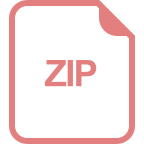














