def Database(file1, file2, file3): # 导入文件内容,建立相关库 with open(file1, 'r', encoding='utf8') as f1: for line in f1.readlines(): line = str(line).split() Plants.extend(line) f1.close() with open(file2, 'r', encoding='utf8') as f2: for line in f2.readlines(): line = str(line).split() Feature.extend(line) f2.close() with open(file3, 'r', encoding='utf8') as f3: for line in f3: line = line.split() Rules.append(line) f3.close()
时间: 2024-01-19 12:05:12 浏览: 64
这段代码是一个名为Database的函数,用于从三个文件中读取数据,建立相关的数据库。
函数接受三个参数file1、file2和file3,分别表示存储植物名称、植物特征和推理规则的文件。函数使用三个with语句打开这三个文件,并使用readlines()方法从文件中读取每一行内容。
对于植物名称和植物特征文件,函数使用一个for循环迭代文件中的每一行,将每一行的内容转换为一个字符串列表,并将这些列表添加到全局变量Plants和Feature中。
对于推理规则文件,函数也使用一个for循环迭代文件中的每一行,将每一行的内容转换为一个字符串列表,并将这些列表添加到全局变量Rules中。
最后,使用close()方法关闭文件。
相关问题
具体解释import mysql.connector import yaml import os def get_mysql_config(): config_file = os.path.join(os.path.dirname(os.path.abspath(__file__)), '..', 'config', 'config.yaml') try: with open(config_file, encoding='utf-8') as f: config = yaml.safe_load(f) return config['mysql'] except IOError: print('文件不存在,新建 config/config.yaml 文件...') config = {'mysql': { 'host': 'localhost', 'port': 3306, 'user': 'root', 'password': 'password', 'database': 'jobs' }} with open('config/config.yaml', 'w') as f: yaml.dump(config, f) return config['mysql']
这段代码主要是用来获取 MySQL 数据库的配置信息。代码首先导入了 `mysql.connector`、`yaml` 和 `os` 模块。然后定义了一个名为 `get_mysql_config()` 的函数,该函数会从配置文件中读取 MySQL 数据库的相关配置信息。具体实现步骤如下:
1. 使用 `os.path.abspath(__file__)` 获取当前文件的绝对路径。
2. 使用 `os.path.dirname()` 获取当前文件所在的目录路径。
3. 使用 `os.path.join()` 拼接出配置文件的绝对路径。
4. 使用 `yaml.safe_load()` 方法读取配置文件并将其转换为 Python 对象。
5. 获取 MySQL 数据库的配置信息,并将其作为函数的返回值。
6. 如果配置文件不存在,则新建一个默认的配置文件,并将其作为函数的返回值。
在这段代码中,`config['mysql']` 是一个字典,包含了 MySQL 数据库的相关配置信息,比如主机名、端口号、用户名、密码和数据库名等。可以根据需要进行修改。
lass MedicalGraph: def __init__(self): cur_dir = '/'.join(os.path.abspath(__file__).split('/')[:-1])#获取当前绝对路径得上级目录 self.data_path = os.path.join(cur_dir, 'data/medical.json')#获取json得路径 self.g = Graph("http://localhost:7474", auth=("neo4j", "15113746199yuan"), name="neo4j")这处代码如何改写成正确的格式
这段代码看起来没有语法错误,但是可能存在以下问题:
1. 如果 `medical.json` 文件不存在或者路径错误,程序会抛出 `FileNotFoundError` 异常。
2. 如果 Neo4j 数据库没有运行或者用户名/密码不正确,程序会抛出 `Neo4jError` 异常。
为了处理这些异常情况,可以在代码中添加异常处理逻辑。以下是一个示例:
```python
class MedicalGraph:
def __init__(self):
cur_dir = '/'.join(os.path.abspath(__file__).split('/')[:-1])
self.data_path = os.path.join(cur_dir, 'data/medical.json')
try:
self.g = Graph("http://localhost:7474", auth=("neo4j", "15113746199yuan"), name="neo4j")
except ServiceUnavailable:
print("Neo4j database is not available.")
except AuthError:
print("Invalid Neo4j username or password.")
except Exception as e:
print("Failed to connect to Neo4j database:", e)
try:
with open(self.data_path, 'r', encoding='utf-8') as f:
self.medical_data = json.load(f)
except FileNotFoundError:
print("Cannot find medical.json file.")
except Exception as e:
print("Failed to load medical.json file:", e)
```
在这个示例中,我们使用了 `try-except` 块来捕获可能出现的异常,并在发生异常时打印错误信息。这样可以帮助我们更好地调试程序,找到问题所在。
阅读全文
相关推荐
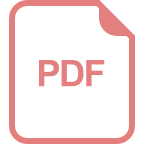
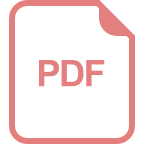
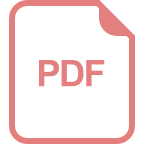





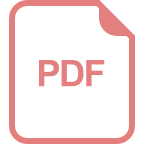
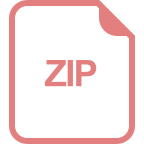
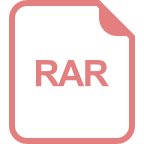
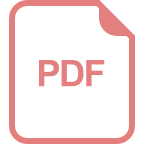
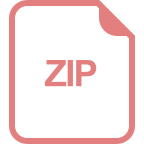
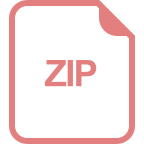
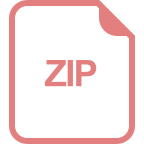
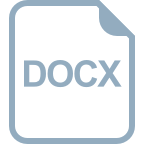