cgcs2000 大地坐标和空间直角坐标转换代码
时间: 2023-11-13 21:06:17 浏览: 47
以下是实现将大地坐标转换为空间直角坐标的 Python 代码:
```python
import math
def geodetic_to_cartesian(latitude, longitude, height):
# WGS-84椭球体参数
a = 6378137.0
f = 1 / 298.257223563
b = a * (1 - f)
e2 = (a ** 2 - b ** 2) / (a ** 2)
# 弧度制转换
lat_rad = math.radians(latitude)
lon_rad = math.radians(longitude)
# 计算卯酉圈半径和子午圈半径
N = a / math.sqrt(1 - e2 * math.sin(lat_rad) ** 2)
M = a * (1 - e2) / math.sqrt((1 - e2 * math.sin(lat_rad) ** 2) ** 3)
# 计算空间直角坐标
x = (N + height) * math.cos(lat_rad) * math.cos(lon_rad)
y = (N + height) * math.cos(lat_rad) * math.sin(lon_rad)
z = (N * (1 - e2) + height) * math.sin(lat_rad)
return x, y, z
```
其中,`latitude`、`longitude`、`height` 分别为输入的纬度、经度和高度。输出为空间直角坐标系下的 x、y、z 坐标。
以下是实现将空间直角坐标转换为大地坐标的 Python 代码:
```python
def cartesian_to_geodetic(x, y, z):
# WGS-84椭球体参数
a = 6378137.0
f = 1 / 298.257223563
b = a * (1 - f)
e2 = (a ** 2 - b ** 2) / (a ** 2)
# 计算经纬度
p = math.sqrt(x ** 2 + y ** 2)
lat_rad = math.atan(z / (p * (1 - e2)))
lat = math.degrees(lat_rad)
lon = math.degrees(math.atan2(y, x))
# 计算高度
N = a / math.sqrt(1 - e2 * math.sin(lat_rad) ** 2)
height = p / math.cos(lat_rad) - N
return lat, lon, height
```
其中,`x`、`y`、`z` 分别为输入的空间直角坐标系下的 x、y、z 坐标。输出为大地坐标系下的纬度、经度和高度。
需要注意的是,上述代码仅适用于 WGS-84 椭球体。如果需要使用其他椭球体,请相应地修改参数。
相关推荐
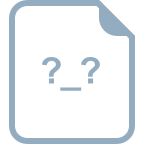














