将许多字符串存放在二叉树中c语言
时间: 2025-01-04 13:38:22 浏览: 8
在C语言中,将许多字符串存放在二叉树(例如二叉搜索树或字典树)中,通常是通过将每个字符串视为一个键,然后根据字符串的字典序(即按字母顺序)来进行组织。以下是基本步骤:
1. **定义节点**:创建一个二叉树节点结构,包含一个指向字符串的指针和两个子节点。
```c
struct Node {
char* key; // 存放字符串的指针
struct Node* left;
struct Node* right;
};
```
2. **比较函数**:为了插入和查找,需要定义一个函数来比较两个字符串(这里假设我们使用标准的字典序)。
```c
int compare_strings(const char* str1, const char* str2) {
while (*str1 && *str2 && *str1 == *str2) {
str1++;
str2++;
}
return *str1 - *str2;
}
```
3. **插入函数**:基于比较函数,我们可以插入新字符串到适当位置。
```c
struct Node* insert(struct Node* node, char* key) {
if (node == NULL) {
node = (struct Node*) malloc(sizeof(struct Node));
node->key = key;
node->left = NULL;
node->right = NULL;
} else if (compare_strings(key, node->key) < 0) {
node->left = insert(node->left, key);
} else {
node->right = insert(node->right, key);
}
return node;
}
```
4. **构建树**:遍历字符串数组并调用insert函数来填充树。
```c
void build_tree(char** strings, int length, struct Node** root) {
*root = insert(NULL, strings[0]);
for (int i = 1; i < length; i++) {
*root = insert(*root, strings[i]);
}
}
```
5. **查询或遍历**:对于查找、删除或其他操作,你可以根据需要实现相应的函数。
阅读全文
相关推荐









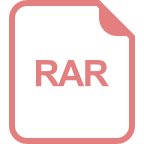
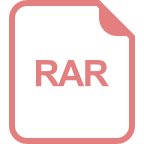
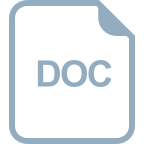





