Katalon8.6.0导入java-diff-util自动化比较两个文件夹。当文件在两个文件夹中都存在但内容不同,则将差异数据的行数和内容输出到html文件中,并将差异字段高亮显示。当文件只在一个文件夹中存在,则将信息输出。要求以文件为单位生成html
时间: 2024-02-27 20:57:22 浏览: 21
好的,以下是一个示例脚本,可以实现您的要求:
```
import org.apache.commons.io.FileUtils;
import difflib.*;
import java.util.List;
import java.io.File;
import java.io.IOException;
import com.kms.katalon.core.annotation.Keyword
import com.kms.katalon.core.util.KeywordUtil
public class DiffUtil {
@Keyword
def compareFolders(String folder1Path, String folder2Path) {
File folder1 = new File(folder1Path)
File folder2 = new File(folder2Path)
if (!folder1.exists() || !folder1.isDirectory()) {
KeywordUtil.markFailed("Folder1 does not exist or is not a directory")
return
}
if (!folder2.exists() || !folder2.isDirectory()) {
KeywordUtil.markFailed("Folder2 does not exist or is not a directory")
return
}
File[] files1 = folder1.listFiles()
File[] files2 = folder2.listFiles()
for (File file1 : files1) {
File file2 = FileUtils.getFile(folder2, file1.getName())
if (file2.exists() && file2.isFile()) {
compareFiles(file1, file2)
} else {
KeywordUtil.logInfo("File " + file1.getName() + " only exists in folder1")
// 输出信息到HTML文件
writeInfoToHtml(file1.getName(), "only exists in folder1")
}
}
for (File file2 : files2) {
File file1 = FileUtils.getFile(folder1, file2.getName())
if (!file1.exists()) {
KeywordUtil.logInfo("File " + file2.getName() + " only exists in folder2")
// 输出信息到HTML文件
writeInfoToHtml(file2.getName(), "only exists in folder2")
}
}
}
@Keyword
def compareFiles(File file1, File file2) {
try {
List<String> lines1 = FileUtils.readLines(file1, "UTF-8")
List<String> lines2 = FileUtils.readLines(file2, "UTF-8")
Patch<String> patch = DiffUtils.diff(lines1, lines2)
if (patch.getDeltas().size() > 0) {
KeywordUtil.logInfo("File " + file1.getName() + " and " + file2.getName() + " are different")
// 输出差异信息到HTML文件
writeDiffToHtml(file1.getName(), patch)
} else {
KeywordUtil.logInfo("File " + file1.getName() + " and " + file2.getName() + " are the same")
}
} catch (IOException e) {
e.printStackTrace()
}
}
def writeInfoToHtml(String fileName, String info) {
KeywordUtil.markWarning(info)
String htmlContent = "<p>" + fileName + ": " + info + "</p>"
writeHtmlFile(fileName, htmlContent)
}
def writeDiffToHtml(String fileName, Patch<String> patch) {
StringBuilder htmlContent = new StringBuilder()
htmlContent.append("<p>" + fileName + ":</p>")
htmlContent.append("<table><tbody>")
for (Delta<String> delta : patch.getDeltas()) {
htmlContent.append("<tr>")
htmlContent.append("<td>" + delta.getOriginal().getPosition() + "</td>")
htmlContent.append("<td>" + delta.getRevised().getPosition() + "</td>")
htmlContent.append("</tr>")
htmlContent.append("<tr>")
htmlContent.append("<td><pre>")
for (Object line : delta.getOriginal().getLines()) {
htmlContent.append("<span class=\"deleted\">" + line + "</span>\n")
}
htmlContent.append("</pre></td>")
htmlContent.append("<td><pre>")
for (Object line : delta.getRevised().getLines()) {
htmlContent.append("<span class=\"added\">" + line + "</span>\n")
}
htmlContent.append("</pre></td>")
htmlContent.append("</tr>")
}
htmlContent.append("</tbody></table>")
writeHtmlFile(fileName, htmlContent.toString())
}
def writeHtmlFile(String fileName, String content) {
String htmlFilePath = "Reports/diff_" + fileName + ".html"
try {
FileUtils.writeStringToFile(new File(htmlFilePath), content, "UTF-8")
} catch (IOException e) {
e.printStackTrace()
}
}
}
```
使用上述脚本,您可以使用`compareFolders`关键字来比较两个文件夹中的文件。该脚本会遍历文件夹中的所有文件,如果文件在两个文件夹中都存在但内容不同,则将差异数据的行数和内容输出到HTML文件中,并将差异字段高亮显示。如果文件只在一个文件夹中存在,则将信息输出到HTML文件。对于每个文件,脚本都会生成一个单独的HTML文件,以便您可以轻松查看比较结果。
请注意,上述脚本使用了`org.apache.commons.io.FileUtils`和`difflib`库。您需要将这些库添加到您的项目中,以便脚本可以正常运行。
相关推荐
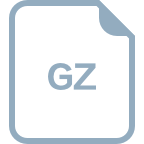
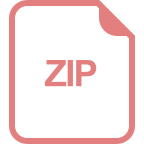
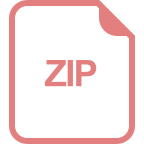






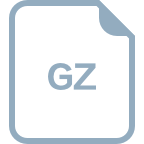
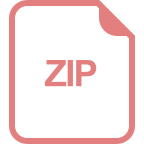
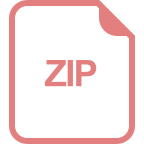
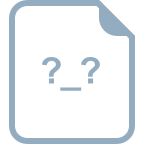
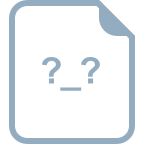
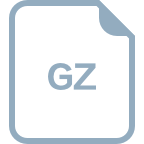
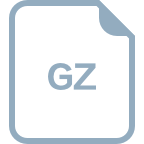
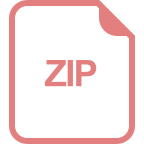
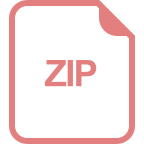
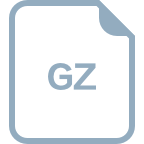