c++ shared_ptr代码实现
时间: 2023-10-17 14:16:47 浏览: 177
下面是一个简单的 shared_ptr 的代码实现:
```cpp
template<typename T>
class shared_ptr {
public:
shared_ptr() : ptr(nullptr), ref_count(nullptr) {}
shared_ptr(T* p) : ptr(p), ref_count(new int(1)) {}
shared_ptr(const shared_ptr& other) : ptr(other.ptr), ref_count(other.ref_count) {
if (ref_count) {
++(*ref_count);
}
}
shared_ptr& operator=(const shared_ptr& other) {
if (this != &other) {
release();
ptr = other.ptr;
ref_count = other.ref_count;
if (ref_count) {
++(*ref_count);
}
}
return *this;
}
~shared_ptr() {
release();
}
T& operator*() const { return *ptr; }
T* operator->() const { return ptr; }
operator bool() const { return ptr != nullptr; }
T* get() const { return ptr; }
private:
void release() {
if (ref_count) {
--(*ref_count);
if (*ref_count == 0) {
delete ptr;
delete ref_count;
}
ptr = nullptr;
ref_count = nullptr;
}
}
T* ptr;
int* ref_count;
};
```
在这个实现中,我们使用一个指针 `ptr` 来保存所指向的对象,使用一个指针 `ref_count` 来保存指向该对象的共享指针数量。当一个新的 shared_ptr 对象创建时,我们将 `ref_count` 初始化为 1,表示当前只有一个 shared_ptr 对象指向该对象。当我们拷贝一个 shared_ptr 对象时,我们将 `ref_count` 增加 1,并与原对象共享同一个指针。当一个 shared_ptr 对象被销毁时,我们将 `ref_count` 减 1,如果此时 `ref_count` 的值为 0,表示没有任何 shared_ptr 对象指向该对象了,我们就可以释放该对象的内存和 `ref_count` 的内存了。
此外,我们还实现了 `operator*` 和 `operator->` 来方便访问所指向的对象,实现了 `operator bool` 来判断 shared_ptr 是否为空,实现了 `get` 函数来获取指向的对象的指针。
阅读全文
相关推荐
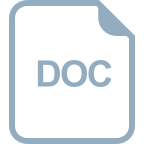
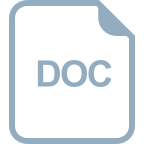
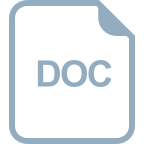
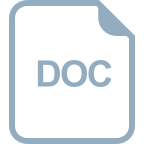
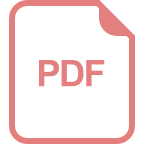
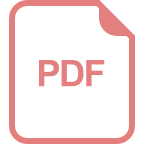
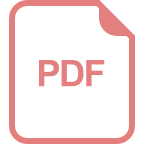
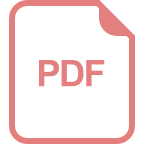
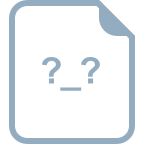
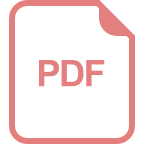
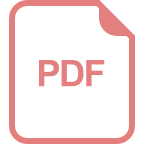
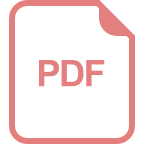
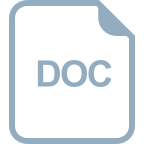
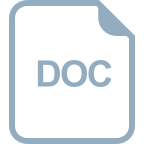
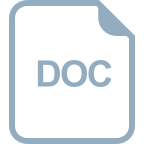
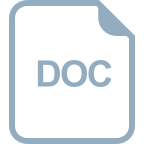


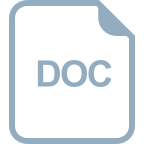