c++实现shared_ptr
时间: 2023-10-05 18:04:06 浏览: 130
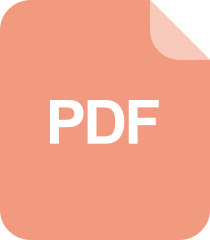
详解C++中shared_ptr的使用教程
shared_ptr是C++中用于动态内存管理的智能指针之一。它能够记录对象被引用的次数,主要用于管理动态创建的对象的销毁。使用shared_ptr可以避免内存泄漏和悬挂指针等问题。
在C++中,实现shared_ptr通常需要以下几个步骤:
1. 定义一个模板类,例如SHARED_ptr,该类将作为智能指针使用。
2. 在SHARED_ptr类中,定义一个指针成员变量ptr,用于指向实际的对象。
3. 在SHARED_ptr类中,定义一个计数器类的指针成员变量refcount,用于记录对象被引用的次数。
4. 在SHARED_ptr类中,实现构造函数,用于初始化指针和计数器。
5. 在SHARED_ptr类中,实现拷贝构造函数和赋值操作符重载,用于处理多个智能指针共享同一对象的情况。
6. 在SHARED_ptr类中,实现析构函数,用于释放对象的内存空间。
7. 在SHARED_ptr类中,实现箭头运算符重载和解引用运算符重载,用于访问对象的成员函数和数据。
8. 在SHARED_ptr类外部,实现计数器类RefCount,用于记录对象被引用的次数,并在引用次数为0时释放对象的内存空间。
实现shared_ptr的详细代码如下所示:
```cpp
template <class T>
class SHARED_ptr {
private:
T* ptr; // 用来指向堆区对象
RefCount<T>* refcount; // 指向计数器对象的指针
public:
SHARED_ptr(T* p) : ptr(p), refcount(new RefCount<T>()) {
refcount->increment();
}
SHARED_ptr(const SHARED_ptr<T>& other) : ptr(other.ptr), refcount(other.refcount) {
refcount->increment();
}
~SHARED_ptr() {
if (refcount->decrement() == 0) {
delete ptr;
delete refcount;
}
}
T* operator->() const {
return ptr;
}
T& operator*() const {
return *ptr;
}
SHARED_ptr& operator=(const SHARED_ptr<T>& other) {
if (this != &other) {
if (refcount->decrement() == 0) {
delete ptr;
delete refcount;
}
ptr = other.ptr;
refcount = other.refcount;
refcount->increment();
}
return *this;
}
};
```
以上是一个简单的C++实现shared_ptr的示例代码。通过使用shared_ptr,我们可以方便地管理动态创建的对象的生命周期,并避免内存泄漏和悬挂指针等问题。
阅读全文
相关推荐
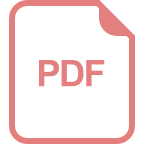
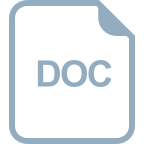


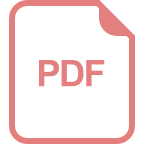
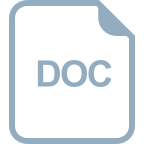










