yplov8 pyqt6后端代码
时间: 2024-12-31 22:40:22 浏览: 8
### PyQt6 后端代码示例
对于希望集成高性能后端处理到 PyQt6 应用程序中的开发者来说,可以采用将核心算法实现于 C/C++ 或者 Rust 中的方法[^3]。下面是一个简单的例子来展示如何编写并利用这些技术。
#### 使用 Pybind11 和 C++
假设有一个名为 `example.cpp` 的文件:
```cpp
#include <pybind11/pybind11.h>
int add(int i, int j) {
return i + j;
}
PYBIND11_MODULE(example, m) {
m.doc() = "Example plugin"; // optional module docstring
m.def("add", &add, "A function which adds two numbers");
}
```
编译上述源码成共享库之后,在Python侧可以通过如下方式加载它并与之交互:
```python
import example # 导入由C++构建的扩展模块
print(f"The sum of 2 and 3 is {example.add(2, 3)}") # 调用C++函数
```
接着可以在 PyQt6 GUI 程序里引入此功能作为后台支持的一部分。
#### 结合 PyQt6 进行前端开发
为了使这个加法器能够在图形界面上工作,这里给出一段简化版的 PyQt6 代码片段用于创建窗口界面,并连接至之前定义好的外部运算逻辑:
```python
from PyQt6.QtWidgets import QApplication, QWidget, QVBoxLayout, QLineEdit, QPushButton, QLabel
class CalculatorWindow(QWidget):
def __init__(self):
super().__init__()
layout = QVBoxLayout()
self.result_label = QLabel('Result will appear here')
layout.addWidget(self.result_label)
self.input_a = QLineEdit()
layout.addWidget(QLabel('Input A'))
layout.addWidget(self.input_a)
self.input_b = QLineEdit()
layout.addWidget(QLabel('Input B'))
layout.addWidget(self.input_b)
calculate_button = QPushButton('Calculate Sum')
calculate_button.clicked.connect(self.on_calculate_clicked)
layout.addWidget(calculate_button)
self.setLayout(layout)
def on_calculate_clicked(self):
try:
a = int(self.input_a.text())
b = int(self.input_b.text())
result = example.add(a, b) # 调用来自C++的add方法
self.result_label.setText(f'Sum: {result}')
except ValueError as e:
self.result_label.setText(str(e))
if __name__ == '__main__':
app = QApplication([])
window = CalculatorWindow()
window.show()
app.exec()
```
这段代码展示了怎样把基于 C++ 实现的功能嵌入到一个简易的计算器应用之中,其中涉及到了基本控件如文本框 (`QLineEdit`) 及按钮(`QPushButton`) 的使用以及信号槽机制的应用。
阅读全文
相关推荐











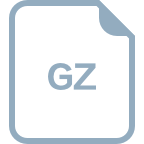


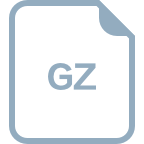
