tkinter实现记事本
时间: 2023-12-03 13:39:45 浏览: 141
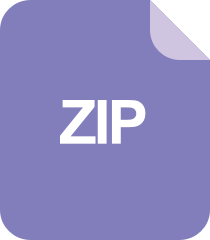
python tkinter写的记事本(代码行数400+,各种tkinter均使用了)

以下是使用Tkinter在Python中创建一个简单的记事本的代码示例:
```python
from tkinter import *
from tkinter.filedialog import *
class Notepad:
__root = Tk()
# 设置默认窗口大小和文本
__thisWidth = 300
__thisHeight = 300
__thisTextArea = Text(__root)
__thisMenuBar = Menu(__root)
__thisFileMenu = Menu(__thisMenuBar, tearoff=0)
__thisEditMenu = Menu(__thisMenuBar, tearoff=0)
__thisHelpMenu = Menu(__thisMenuBar, tearoff=0)
# 添加文件菜单项
__thisFileMenu.add_command(label="New", command=__newFile)
__thisFileMenu.add_command(label="Open", command=__openFile)
__thisFileMenu.add_command(label="Save", command=__saveFile)
__thisFileMenu.add_separator()
__thisFileMenu.add_command(label="Exit", command=__quitApplication)
__thisMenuBar.add_cascade(label="File", menu=__thisFileMenu)
# 添加编辑菜单项
__thisEditMenu.add_command(label="Cut", command=__cut)
__thisEditMenu.add_command(label="Copy", command=__copy)
__thisEditMenu.add_command(label="Paste", command=__paste)
__thisMenuBar.add_cascade(label="Edit", menu=__thisEditMenu)
# 添加帮助菜单项
__thisHelpMenu.add_command(label="About Notepad", command=__showAbout)
__thisMenuBar.add_cascade(label="Help", menu=__thisHelpMenu)
__root.config(menu=__thisMenuBar)
__thisScrollBar = Scrollbar(__thisTextArea)
__file = None
def __init__(self, **kwargs):
# 设置窗口大小
try:
self.__thisWidth = kwargs['width']
except KeyError:
pass
try:
self.__thisHeight = kwargs['height']
except KeyError:
pass
# 设置窗口标题
self.__root.title("Untitled - Notepad")
# 设置窗口的初始大小
screenWidth = self.__root.winfo_screenwidth()
screenHeight = self.__root.winfo_screenheight()
# 计算窗口的左上角坐标
left = (screenWidth / 2) - (self.__thisWidth / 2)
top = (screenHeight / 2) - (self.__thisHeight / 2)
# 设置窗口的尺寸和位置
self.__root.geometry('%dx%d+%d+%d' % (self.__thisWidth, self.__thisHeight, left, top))
# 添加文本区域和滚动条
self.__root.grid_rowconfigure(0, weight=1)
self.__root.grid_columnconfigure(0, weight=1)
self.__thisTextArea.grid(sticky=N + E + S + W)
self.__thisTextArea.configure(yscrollcommand=self.__thisScrollBar.set)
self.__thisScrollBar.grid(row=0, column=1, sticky=N + S + E)
self.__thisScrollBar.configure(command=self.__thisTextArea.yview)
def __quitApplication(self):
self.__root.destroy()
def __showAbout(self):
showinfo("Notepad", "Created by: Your Name")
def __openFile(self):
self.__file = askopenfilename(defaultextension=".txt", filetypes=[("All Files", "*.*"), ("Text Documents", "*.txt")])
if self.__file == "":
self.__file = None
else:
self.__root.title(os.path.basename(self.__file) + " - Notepad")
self.__thisTextArea.delete(1.0, END)
file = open(self.__file, "r")
self.__thisTextArea.insert(1.0, file.read())
file.close()
def __newFile(self):
self.__root.title("Untitled - Notepad")
self.__file = None
self.__thisTextArea.delete(1.0, END)
def __saveFile(self):
if self.__file == None:
self.__file = asksaveasfilename(initialfile='Untitled.txt', defaultextension=".txt", filetypes=[("All Files", "*.*"), ("Text Documents", "*.txt")])
if self.__file == "":
self.__file = None
else:
file = open(self.__file, "w")
file.write(self.__thisTextArea.get(1.0, END))
file.close()
self.__root.title(os.path.basename(self.__file) + " - Notepad")
else:
file = open(self.__file, "w")
file.write(self.__thisTextArea.get(1.0, END))
file.close()
def __cut(self):
self.__thisTextArea.event_generate("<<Cut>>")
def __copy(self):
self.__thisTextArea.event_generate("<<Copy>>")
def __paste(self):
self.__thisTextArea.event_generate("<<Paste>>")
def run(self):
self.__root.mainloop()
notepad = Notepad(width=600, height=400)
notepad.run()
```
阅读全文
相关推荐
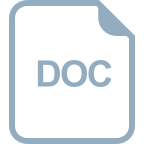
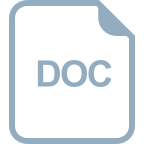

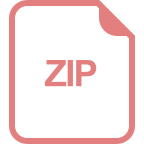
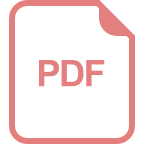



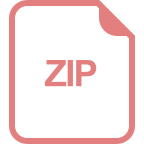
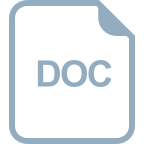
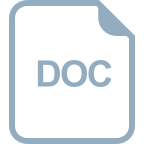




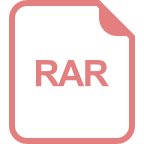