如何编写一个函数,接受输入为特定年份、月份和日期,然后计算并返回该日期在当年所对应的天数?
时间: 2024-11-20 11:40:58 浏览: 68
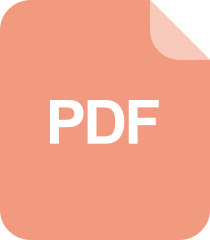
php计算两个日期时间差(返回年、月、日)
为了编写一个计算特定年份、月份和日期在当年对应天数的函数,你可以按照以下步骤设计:
1. 确定基础数据结构:
创建一个包含全年的天数列表,考虑到闰年和平年的区别。例如,非闰年的2月只有28天,而闰年的2月有29天。
2. 定义函数:
使用一个名为`calculate_days`的函数,接收三个参数:`year`, `month`, 和 `day`。函数内部可以先检查闰年情况,再累加之前的月份天数。
3. 编写计算逻辑:
a) 判断是否为闰年,通常使用模运算 (`if (year % 4 == 0 and year % 100 != 0) || (year % 400 == 0)`)。
b) 初始化一个计数器(比如`total_days`),通常设置为1,表示年初的第一天。
c) 遍历从1月到目标月的月份,将每个月份的天数累加到`total_days`上。
d) 最后,把目标月的剩余天数加上。
4. 返回结果:
函数执行完毕后,返回`total_days`作为结果。
下面是使用 Python 实现的一个简单示例:
```python
def calculate_days(year, month, day):
days_in_month = [0, 31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
if calendar.isleap(year): # 判断是否闰年
days_in_month[1] = 29
total_days = 0
for i in range(1, month):
total_days += days_in_month[i]
total_days += day
return total_days
# 测试
year = 2023
month = 2
day = 24
print(calculate_days(year, month, day)) # 输出:57
```
阅读全文
相关推荐
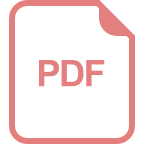
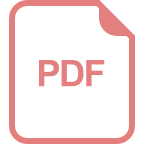
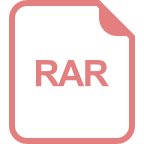
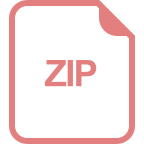
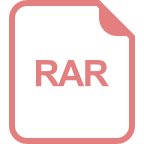
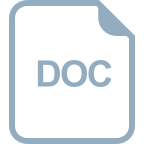
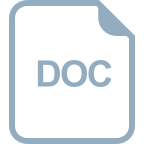
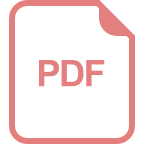
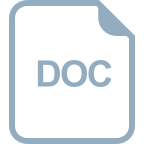
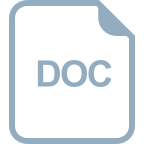
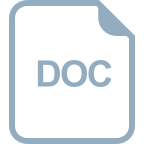
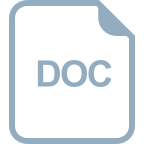
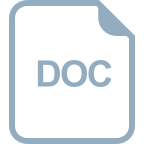
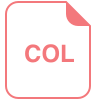


