java yyyy-MM-dd HH:mm转变yyyy-MM-dd HH:mm:ss
时间: 2024-06-13 08:05:26 浏览: 198
可以使用SimpleDateFormat类将时间格式从"yyyy-MM-dd HH:mm"转换为"yyyy-MM-dd HH:mm:ss"。具体实现方法如下所示:
```java
String testDate = "2023-01-05 15:15";SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm");
Date date = sdf.parse(testDate);
sdf.applyPattern("yyyy-MM-dd HH:mm:ss");
String newDate = sdf.format(date);
```
以上代码中,首先定义了一个时间字符串"testDate",然后使用SimpleDateFormat类将其转换为Date类型的时间对象"date"。接着,使用applyPattern()方法将SimpleDateFormat对象的时间格式修改为"yyyy-MM-dd HH:mm:ss",最后使用format()方法将时间对象转换为字符串类型的时间"newDate"。
相关问题
java yyyy-MM-dd HH:mm 转 yyyy-MM-dd HH:mm:ss
你使用 SimpleDateFormat 类来实现这个转换。下面是一个示例代码:
```java
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
String dateTime = "2022-02-01 12:34";
String convertedDateTime = convertDateTime(dateTime);
System.out.println(convertedDateTime);
}
public static String convertDateTime(String dateTime) {
SimpleDateFormat inputFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm");
SimpleDateFormat outputFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
try {
Date date = inputFormat.parse(dateTime);
return outputFormat.format(date);
} catch (ParseException e) {
e.printStackTrace();
}
return null;
}
}
```
在上面的代码中,我们通过使用 SimpleDateFormat 类,先定义了输入格式("yyyy-MM-dd HH:mm")和输出格式("yyyy-MM-dd HH:mm:ss")。然后,我们使用 parse() 方法将输入的日期时间字符串解析为 Date 对象,再使用 format() 方法将 Date 对象格式化为指定的输出格式。最后,将转换后的日期时间字符串返回。
在示例中,输入的日期时间是 "2022-02-01 12:34",经过转换后输出的日期时间是 "2022-02-01 12:34:00"。你可以根据需要修改输入和输出的日期时间字符串。
java yyyy-MM-dd HH:mm 自动补全 yyyy-MM-dd HH:mm:ss
你可以使用`java.time.LocalDateTime`类来处理日期和时间,并且可以使用`DateTimeFormatter`来格式化日期和时间。以下是一个示例代码,可以将输入的日期和时间自动补全到秒:
```java
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateTimeAutoComplete {
public static void main(String[] args) {
String input = "2022-01-01 10:30";
DateTimeFormatter inputFormatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm");
LocalDateTime dateTime = LocalDateTime.parse(input, inputFormatter);
DateTimeFormatter outputFormatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
String output = dateTime.format(outputFormatter);
System.out.println(output); // 输出:2022-01-01 10:30:00
}
}
```
在上面的示例中,我们首先定义了输入的日期和时间格式("yyyy-MM-dd HH:mm"),然后使用`LocalDateTime.parse()`方法将输入字符串解析为`LocalDateTime`对象。接下来,我们定义了输出的日期和时间格式("yyyy-MM-dd HH:mm:ss"),并使用`dateTime.format()`方法将`LocalDateTime`对象格式化为字符串。最后,我们打印输出的结果。
阅读全文
相关推荐
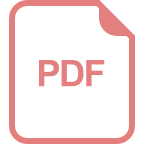
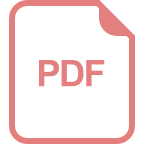













