tls_client是什么
时间: 2023-08-13 07:06:32 浏览: 243
tls_client是Transport Layer Security (TLS)协议的客户端实现。TLS是一种加密协议,用于保护网络通信的安全性和隐私性。TLS协议可以在客户端和服务器之间建立安全通信通道,并对数据进行加密和解密,以确保数据传输的机密性和完整性。tls_client作为TLS协议的客户端实现,负责与TLS服务器进行握手和建立安全通道,从而实现安全的通信。
相关问题
STREAM_CRYPTO_METHOD_TLS_CLIENT 等于57 stream_socket_enable_crypto无响应
`STREAM_CRYPTO_METHOD_TLS_CLIENT` 是用于在流上启用 TLS 客户端加密的常量,它的值为57。而 `stream_socket_enable_crypto` 函数是用于在流上启用加密的函数,可以使用它来启用 TLS 加密。
如果 `stream_socket_enable_crypto` 函数无响应,可能是因为与服务器的握手失败,或者由于服务器证书无法验证而导致连接失败。您可以尝试检查服务器证书是否有效,并确保您正在使用正确的端口和协议。另外,您还可以尝试在调用 `stream_socket_enable_crypto` 函数之前,先建立好与服务器的连接。
写一个基于mbed TLS实现"MBEDTLS_TLS_DHE_RSA_WITH_AES_128_GCM_SHA256"cipher suite的示例代码
以下是基于mbed TLS实现"MBEDTLS_TLS_DHE_RSA_WITH_AES_128_GCM_SHA256"cipher suite的示例代码:
```c
#include "mbedtls/config.h"
#include "mbedtls/platform.h"
#include "mbedtls/entropy.h"
#include "mbedtls/ctr_drbg.h"
#include "mbedtls/ssl.h"
#include "mbedtls/net_sockets.h"
#include "mbedtls/error.h"
#include "mbedtls/x509_crt.h"
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SERVER_NAME "www.example.com"
#define SERVER_PORT "443"
int main(int argc, char *argv[])
{
int ret;
mbedtls_entropy_context entropy;
mbedtls_ctr_drbg_context ctr_drbg;
mbedtls_ssl_context ssl;
mbedtls_ssl_config ssl_conf;
mbedtls_x509_crt cacert;
mbedtls_net_context server_fd;
char buf[1024];
const char *pers = "mbedtls_tls_dhe_rsa_with_aes_128_gcm_sha256";
mbedtls_ssl_init(&ssl);
mbedtls_ssl_config_init(&ssl_conf);
mbedtls_ctr_drbg_init(&ctr_drbg);
mbedtls_entropy_init(&entropy);
mbedtls_x509_crt_init(&cacert);
// Initialize the entropy pool and the random number generator
if ((ret = mbedtls_entropy_add_source(&entropy, NULL, 0, MBEDTLS_ENTROPY_SOURCE_STRONG)) != 0) {
printf("mbedtls_entropy_add_source returned %d\n", ret);
goto exit;
}
if ((ret = mbedtls_ctr_drbg_seed(&ctr_drbg, mbedtls_entropy_func, &entropy, (const unsigned char *) pers, strlen(pers))) != 0) {
printf("mbedtls_ctr_drbg_seed returned %d\n", ret);
goto exit;
}
// Load the trusted CA certificates
if ((ret = mbedtls_x509_crt_parse_file(&cacert, "ca.crt")) != 0) {
printf("mbedtls_x509_crt_parse_file returned %d\n", ret);
goto exit;
}
// Initialize the SSL/TLS context and configure it for client use
if ((ret = mbedtls_ssl_config_defaults(&ssl_conf, MBEDTLS_SSL_IS_CLIENT, MBEDTLS_SSL_TRANSPORT_STREAM, MBEDTLS_SSL_PRESET_DEFAULT)) != 0) {
printf("mbedtls_ssl_config_defaults returned %d\n", ret);
goto exit;
}
mbedtls_ssl_conf_authmode(&ssl_conf, MBEDTLS_SSL_VERIFY_REQUIRED);
mbedtls_ssl_conf_ca_chain(&ssl_conf, &cacert, NULL);
mbedtls_ssl_conf_rng(&ssl_conf, mbedtls_ctr_drbg_random, &ctr_drbg);
mbedtls_ssl_conf_ciphersuites(&ssl_conf, mbedtls_ssl_list_ciphersuites());
// Set up the SSL/TLS context for the connection to the server
if ((ret = mbedtls_ssl_setup(&ssl, &ssl_conf)) != 0) {
printf("mbedtls_ssl_setup returned %d\n", ret);
goto exit;
}
if ((ret = mbedtls_ssl_set_hostname(&ssl, SERVER_NAME)) != 0) {
printf("mbedtls_ssl_set_hostname returned %d\n", ret);
goto exit;
}
// Connect to the server
if ((ret = mbedtls_net_connect(&server_fd, SERVER_NAME, SERVER_PORT, MBEDTLS_NET_PROTO_TCP)) != 0) {
printf("mbedtls_net_connect returned %d\n", ret);
goto exit;
}
mbedtls_ssl_set_bio(&ssl, &server_fd, mbedtls_net_send, mbedtls_net_recv, NULL);
// Perform the SSL/TLS handshake with the server
while ((ret = mbedtls_ssl_handshake(&ssl)) != 0) {
if (ret != MBEDTLS_ERR_SSL_WANT_READ && ret != MBEDTLS_ERR_SSL_WANT_WRITE) {
printf("mbedtls_ssl_handshake returned %d\n", ret);
goto exit;
}
}
// Send a request to the server
sprintf(buf, "GET / HTTP/1.1\r\nHost: %s\r\n\r\n", SERVER_NAME);
if ((ret = mbedtls_ssl_write(&ssl, (const unsigned char *) buf, strlen(buf))) != strlen(buf)) {
printf("mbedtls_ssl_write returned %d\n", ret);
goto exit;
}
// Receive the response from the server
do {
memset(buf, 0, sizeof(buf));
ret = mbedtls_ssl_read(&ssl, (unsigned char *) buf, sizeof(buf) - 1);
if (ret == MBEDTLS_ERR_SSL_WANT_READ || ret == MBEDTLS_ERR_SSL_WANT_WRITE) {
continue;
}
if (ret <= 0) {
break;
}
printf("%s", buf);
} while (1);
exit:
mbedtls_x509_crt_free(&cacert);
mbedtls_ssl_config_free(&ssl_conf);
mbedtls_ssl_free(&ssl);
mbedtls_ctr_drbg_free(&ctr_drbg);
mbedtls_entropy_free(&entropy);
mbedtls_net_free(&server_fd);
return ret;
}
```
注意:在运行该示例代码前,需要将ca.crt替换为实际的CA证书文件。
阅读全文
相关推荐
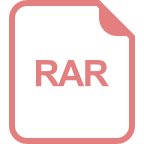
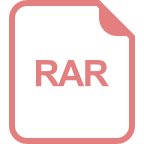
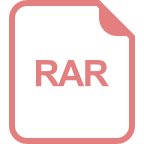

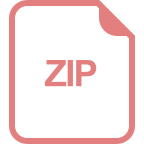
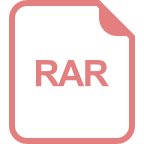
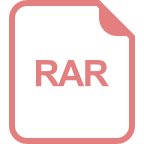
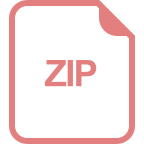
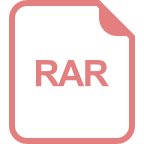
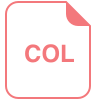
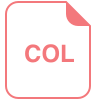




