scaler = StandardScaler(),对三维数组(n_samples, n_time_steps, n_features)转换为2维数组进行标准化,将标准化后的数组还原为3维数组
时间: 2024-03-27 10:41:42 浏览: 21
可以按照以下步骤将三维数组标准化为二维数组:
```python
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
# 假设 X 是你的 3D 数组,形状为 (n_samples, n_time_steps, n_features)
n_samples, n_time_steps, n_features = X.shape
# 将 X 转换为 2D 数组
X_2d = X.reshape(n_samples * n_time_steps, n_features)
# 对 X_2d 进行标准化
X_2d_scaled = scaler.fit_transform(X_2d)
```
然后,可以按照以下步骤将标准化后的二维数组还原为三维数组:
```python
# 将标准化后的 2D 数组还原为原始 2D 数组
X_2d_unscaled = scaler.inverse_transform(X_2d_scaled)
# 将 2D 数组转换回 3D 数组
X_unscaled = X_2d_unscaled.reshape(n_samples, n_time_steps, n_features)
```
在还原过程中,一定要使用和标准化时一样的`scaler`进行逆变换。
相关问题
scaler = StandardScaler(),对三维数组(n_samples, n_time_steps, n_features)转换为2维数组进行标准化,还原为3维数组
可以通过以下方式将三维数组标准化为二维数组:
```python
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
# assume X is your 3D array with shape (n_samples, n_time_steps, n_features)
n_samples, n_time_steps, n_features = X.shape
# reshape X to 2D array
X_2d = X.reshape(n_samples * n_time_steps, n_features)
# standardize X_2d
X_2d_scaled = scaler.fit_transform(X_2d)
```
然后,可以通过以下方式将二维数组还原为三维数组:
```python
# inverse transform to get the original 2D array
X_2d_unscaled = scaler.inverse_transform(X_2d_scaled)
# reshape to the original 3D array
X_unscaled = X_2d_unscaled.reshape(n_samples, n_time_steps, n_features)
```
注意,在还原过程中,一定要使用和标准化时一样的`scaler`进行逆变换。
scaler = StandardScaler(),对三维数组(n_samples, n_time_steps, n_features)标准化
如果你的三维数组的形状为 `(n_samples, n_time_steps, n_features)`,你可以按以下方式在每个特征维度上对其进行标准化:
```python
from sklearn.preprocessing import StandardScaler
import numpy as np
# 创建 StandardScaler 对象并对数据进行标准化
scaler = StandardScaler()
# 对每个特征维度和时间步长分别进行标准化
for i in range(n_features):
for j in range(n_time_steps):
# 获取当前特征维度和时间步长的数据
data = X[:, j, i].reshape(-1, 1)
# 对数据进行标准化
scaler.fit(data)
X[:, j, i] = scaler.transform(data).reshape(-1,)
```
这里我们使用了两个 for 循环,分别迭代每个特征维度和时间步长,然后对每个特征维度和时间步长的数据进行标准化。对于每个特征维度和时间步长,我们首先使用 `X[:, j, i]` 语句获取该数据,然后将其转换为二维数组,并使用 `StandardScaler` 对象对其进行拟合和转换。最后,我们将数据重新赋值给 `X[:, j, i]`,并将其形状转换为一维数组。
需要注意的是,这种方法假设每个特征维度和时间步长之间没有相关性。如果你的数据在特征维度和时间步长之间具有相关性,你可能需要使用其他方法来对其进行预处理。
相关推荐
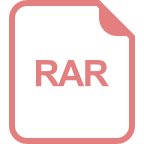
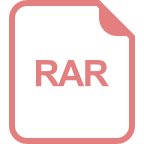













